Bash scripting: an overview
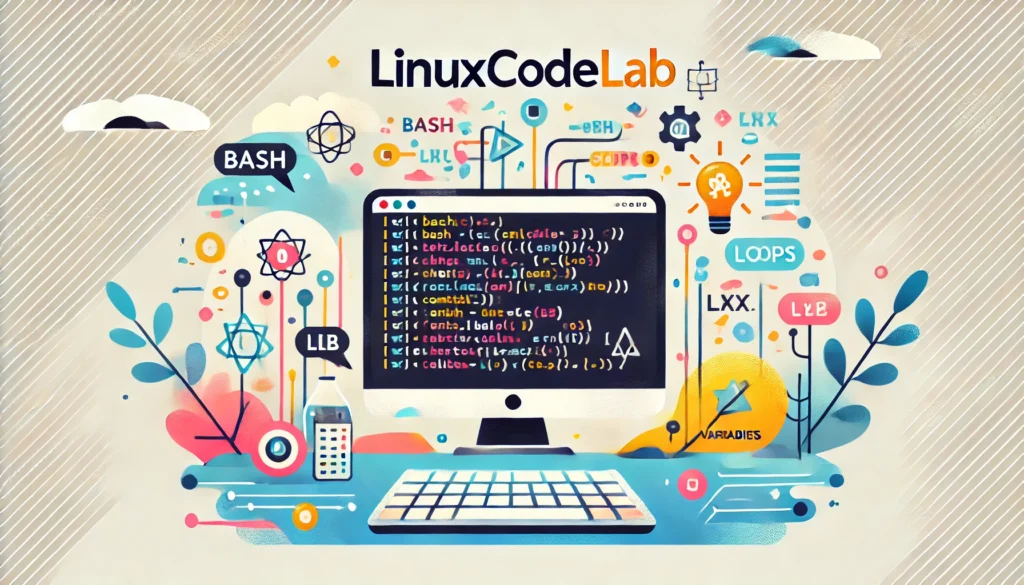
Introduction to bash scripting
Bash, short for “Bourne Again Shell,” is a Unix shell and command language. It’s widely used in Linux and macOS environments. A shell script is a file containing a series of commands that the shell executes. Bash scripting allows you to automate tasks, manipulate files, and configure systems. It’s an essential skill for system administrators, developers, and power users.
The history and significance of bash
Bash was developed by Brian Fox in 1989 as a free software replacement for the Bourne Shell (sh). Over time, it became the default shell on most Linux distributions. Bash provides powerful features, such as command substitution, variables, loops, and conditionals. These features make it a versatile tool for scripting and automation. Knowing bash scripting is invaluable for managing Unix-like systems efficiently.
Understanding bash syntax and commands
Bash scripts are plain text files that start with a shebang (#!
) followed by the path to the interpreter. For bash scripts, this is usually #!/bin/bash
. This line tells the system to execute the script using the bash shell. Below the shebang, you can write a series of commands as you would in the terminal.
Basic syntax
Every bash script begins with the shebang. The rest of the script contains commands, comments, and variables. For example:
bashCopy code#!/bin/bash
echo "Hello, World!"
In this script, echo
is a command that prints text to the terminal. When you run this script, it will display “Hello, World!” on the screen.
Variables in bash
Variables are used to store data that can be referenced later in the script. In bash, you can create a variable by assigning a value to it without spaces around the equal sign. For example:
bashCopy code#!/bin/bash
greeting="Hello"
name="Alice"
echo "$greeting, $name!"
Here, greeting
and name
are variables. The $
symbol is used to access the value of a variable. The script will output “Hello, Alice!”.
Comments
Comments are lines in a script that are not executed. They are used to explain the code and improve readability. In bash, comments start with the #
symbol. For example:
bashCopy code#!/bin/bash
# This is a comment
echo "This will be printed"
Comments help you and others understand what the script does.
Control structures in bash
Control structures allow you to control the flow of a bash script. They include conditional statements, loops, and case statements.
Conditional statements: if-else
Conditional statements let you execute different code based on certain conditions. The basic syntax for an if-else
statement in bash is:
bashCopy code#!/bin/bash
if [ condition ]; then
# Code to execute if condition is true
else
# Code to execute if condition is false
fi
For example:
bashCopy code#!/bin/bash
age=18
if [ $age -ge 18 ]; then
echo "You are an adult."
else
echo "You are a minor."
fi
This script checks if the variable age
is greater than or equal to 18. If true, it prints “You are an adult.” Otherwise, it prints “You are a minor.”
Loops in bash: for, while, until
Loops allow you to repeat a block of code multiple times. Bash supports several types of loops, including for
, while
, and until
.
The for
loop
A for
loop iterates over a list of items. Here’s an example:
bashCopy code#!/bin/bash
for i in 1 2 3 4 5; do
echo "Number: $i"
done
This script prints the numbers 1 through 5.
The while
loop
A while
loop repeats as long as a condition is true:
bashCopy code#!/bin/bash
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
((count++))
done
This script increments the count
variable from 1 to 5 and prints each value.
The until
loop
An until
loop is the opposite of a while
loop. It repeats until a condition becomes true:
bashCopy code#!/bin/bash
count=1
until [ $count -gt 5 ]; do
echo "Count: $count"
((count++))
done
This script does the same as the while
loop but uses an until
loop.
The case
statement
The case
statement is similar to a switch-case statement in other programming languages. It allows you to execute different code based on the value of a variable:
bashCopy code#!/bin/bash
fruit="apple"
case $fruit in
"apple")
echo "You chose apple." ;;
"banana")
echo "You chose banana." ;;
*)
echo "Unknown fruit." ;;
esac
This script checks the value of fruit
and prints a corresponding message.
Working with files and directories
Bash scripting is often used to manage files and directories. Common tasks include creating, deleting, copying, and moving files.
Creating and deleting files
You can create a file using the touch
command and delete it with the rm
command:
bashCopy code#!/bin/bash
touch myfile.txt
echo "File created."
rm myfile.txt
echo "File deleted."
This script creates a file named myfile.txt
and then deletes it.
Copying and moving files
The cp
command copies files, and the mv
command moves them:
bashCopy code#!/bin/bash
cp original.txt copy.txt
echo "File copied."
mv copy.txt moved.txt
echo "File moved."
This script copies original.txt
to copy.txt
and then renames copy.txt
to moved.txt
.
Working with directories
You can create a directory with mkdir
and remove it with rmdir
:
bashCopy code#!/bin/bash
mkdir mydir
echo "Directory created."
rmdir mydir
echo "Directory deleted."
This script creates and deletes a directory named mydir
.
Functions in bash scripting
Functions are reusable blocks of code that you can define and call in a script. They help organize your code and avoid repetition.
Defining and calling functions
You can define a function using the following syntax:
bashCopy code#!/bin/bash
my_function() {
echo "Hello from the function!"
}
my_function
This script defines a function called my_function
and then calls it. The function prints “Hello from the function!”.
Passing arguments to functions
Functions can accept arguments, allowing you to pass data to them:
bashCopy code#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "Alice"
This script defines a function called greet
that accepts one argument. It prints “Hello, Alice!”.
Returning values from functions
You can return a value from a function using the return
statement:
bashCopy code#!/bin/bash
add() {
result=$(($1 + $2))
return $result
}
add 5 3
echo "Sum: $?"
This script defines a function called add
that adds two numbers and returns the result. The $?
variable contains the return value of the last executed command.
Error handling in bash scripting
Error handling is crucial in bash scripting to ensure your scripts behave as expected in all scenarios.
Checking exit status
Every command in bash returns an exit status. A status of 0
indicates success, while any other value indicates failure. You can check the exit status using the $?
variable:
bashCopy code#!/bin/bash
mkdir mydir
if [ $? -eq 0 ]; then
echo "Directory created successfully."
else
echo "Failed to create directory."
fi
This script checks if the mkdir
command succeeded and prints a message accordingly.
Using set -e
for error handling
You can use set -e
to make your script exit immediately if any command fails:
bashCopy code#!/bin/bash
set -e
mkdir mydir
cp nonexistentfile.txt mydir/
This script will exit immediately if the cp
command fails because nonexistentfile.txt
doesn’t exist.
Trapping errors
You can use trap
to execute commands when a script exits or encounters an error:
bashCopy code#!/bin/bash
trap 'echo "An error occurred. Exiting..."; exit 1' ERR
mkdir mydir
cp nonexistentfile.txt mydir/
This script traps any error, prints an error message, and exits with a status of 1
.
Advanced bash scripting techniques
As you become more proficient with bash scripting, you can explore advanced techniques such as arrays, string manipulation, and script debugging.
Working with arrays
Arrays in bash allow you to store multiple values in a single variable:
bashCopy code#!/bin/bash
fruits=("apple" "banana" "cherry")
echo "First fruit: ${fruits[0]}"
echo "All fruits: ${fruits[@]}"
This script defines an array of fruits and prints the first fruit and all fruits.
String manipulation
Bash provides various tools for manipulating strings, such as extracting substrings and replacing text:
bashCopy code#!/bin/bash
text="Hello, World!"
echo "Substring: ${text:7:5}"
echo "Replaced: ${text/World/Bash}"
This script extracts a substring from text
and replaces “World” with “Bash”.
Debugging bash scripts
You can debug bash scripts by running them with the -x
option, which prints each command before executing it:
bashCopy code#!/bin/bash -x
echo "Debugging script..."
This script will print each command as it’s executed, helping you debug the script.
Conclusion
Bash scripting is a powerful tool that can automate tasks, manage systems, and streamline your workflow. With a solid understanding of bash syntax, control structures, file management, functions, and error handling, you can write scripts to solve a wide range of problems. Whether you’re a beginner or an experienced user, mastering bash scripting will enhance your productivity and give you greater control over your Unix-like environments.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut