Command combinations in Linux
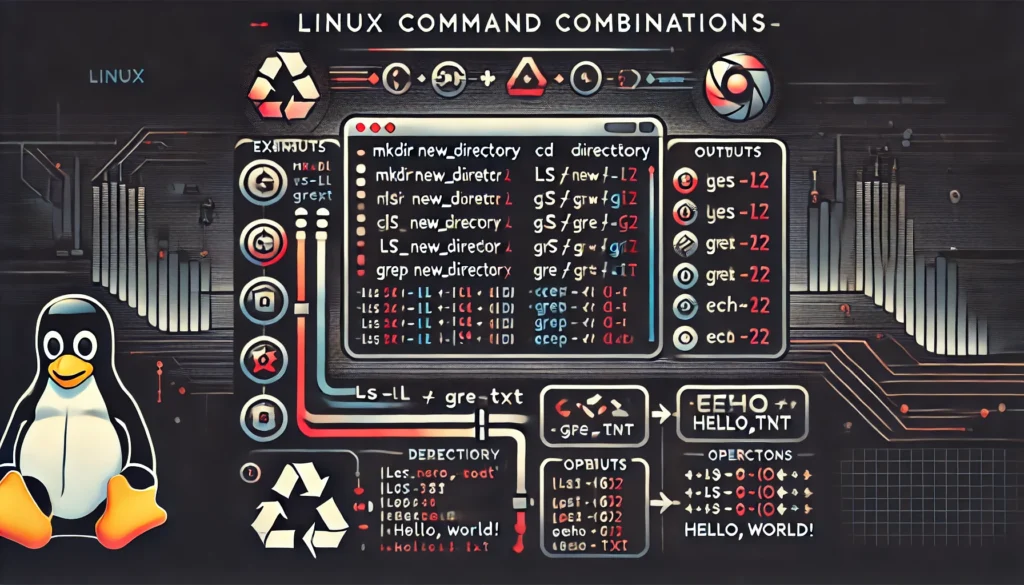
Linux is a powerful and versatile operating system, widely known for its flexibility and command-line interface. Mastering Linux often means becoming proficient with its commands, which can be combined in various ways to achieve more complex tasks. Command combinations, also known as “command chaining,” allow users to string together multiple commands, enhancing efficiency and productivity.
In this article, we will explore some of the most common methods for combining commands in Linux. These include using logical operators, pipelines, subshells, and more. By the end, you’ll have a solid understanding of how to chain commands together, making your Linux experience smoother and more powerful.
1. Command Chaining with Logical Operators
Logical operators in Linux allow you to execute commands conditionally, based on the success or failure of previous commands. The two most commonly used logical operators are the AND (&&
) and OR (||
) operators.
AND Operator (&&
)
The AND operator executes the second command only if the first command succeeds. Success in Linux generally means the command returns an exit status of 0
. If the first command fails, the second command will not run.
Example:
mkdir new_directory && cd new_directory
In this example, the directory new_directory
is created, and if successful, the command changes into that directory. If the mkdir
command fails, cd
will not execute.
OR Operator (||
)
The OR operator runs the second command only if the first command fails. This operator is useful for executing an alternative command when the initial command does not succeed.
Example:
cd existing_directory || echo "Directory does not exist."
Here, if the cd
command fails (perhaps because the directory doesn’t exist), the message “Directory does not exist.” is printed.
2. Using Semicolons (;
) to Chain Commands
The semicolon (;
) is one of the simplest ways to chain commands. It allows you to execute multiple commands sequentially, regardless of the success or failure of the previous command.
Example:
echo "Hello, World!"; ls; pwd
This sequence will print “Hello, World!”, list the files in the current directory, and then display the current working directory. Each command runs independently of the others.
3. Pipelines (|
): Redirecting Output Between Commands
Pipelines (|
) are used to take the output of one command and use it as the input for another command. This method is extremely powerful for processing and filtering data in Linux.
Example:
ls -l | grep ".txt"
In this example, the ls -l
command lists files in long format, and the output is passed to grep
, which filters and displays only the lines containing .txt
, indicating text files.
Pipelines are useful when working with large amounts of data or when you need to perform multiple operations on the output of a command.
4. Subshells: Grouping Commands Together
A subshell is a separate instance of the shell that allows you to group commands together. Commands in a subshell are executed in their own environment, isolated from the parent shell.
Example:
(cd /var/log && ls)
In this example, the commands inside the parentheses are executed in a subshell. The shell changes to /var/log
and lists the files there. After the subshell finishes, the current shell remains in the original directory.
5. Redirection: Input and Output Management
Redirection allows you to control where the output of a command goes or where input comes from. This technique is essential for managing files and data streams in Linux.
Output Redirection (>
and >>
)
The >
operator redirects the output of a command to a file, overwriting the file if it exists. The >>
operator appends the output to the end of a file instead of overwriting it.
Example:
echo "This is a test." > testfile.txt
This command writes “This is a test.” to testfile.txt
, creating the file if it doesn’t exist or overwriting it if it does.
echo "Appending text." >> testfile.txt
This command appends “Appending text.” to the end of testfile.txt
.
Input Redirection (<
)
Input redirection allows a command to take input from a file rather than from the keyboard or another input device.
Example:
sort < unsorted_list.txt
This command reads the contents of unsorted_list.txt
and sorts it, displaying the sorted output.
Combining Redirection with Command Chaining
You can combine redirection with other command chaining techniques for more powerful command sequences.
Example:
grep "error" logfile.txt > errors.txt && cat errors.txt
This command searches for the word “error” in logfile.txt
, writes the matches to errors.txt
, and then displays the contents of errors.txt
if the grep command is successful.
6. The xargs
Command: Building Command Lines from Input
The xargs
command is used to build and execute command lines from standard input. It is often used with commands like find
and grep
to perform actions on files that meet certain criteria.
Example:
find . -name "*.txt" | xargs rm
This command finds all .txt
files in the current directory and its subdirectories, then deletes them using rm
.
7. Command Substitution: Using Command Output as Arguments
Command substitution allows you to use the output of one command as the argument of another command. This technique is essential for dynamic command generation.
There are two ways to perform command substitution in Linux:
- Using backticks (“):
- Using the
$(...)
syntax (recommended)
Example:
files=$(ls *.txt) echo "Text files: $files"
In this example, the output of the ls *.txt
command (which lists all .txt
files) is stored in the files
variable. Then, the echo command displays the list of text files.
8. Conditional Execution: if
Statements in the Shell
Conditional execution with if
statements allows you to perform actions based on the success or failure of commands.
Example:
if grep -q "error" logfile.txt; then echo "Errors found." else echo "No errors." fi
In this script, the grep
command searches for the word “error” in logfile.txt
. If it finds a match, the script prints “Errors found.” Otherwise, it prints “No errors.”
9. Loops: Repeating Commands
Loops are used to repeat commands multiple times or over a range of items. The for
, while
, and until
loops are commonly used in shell scripting.
for
Loop
The for
loop iterates over a list of items and executes commands for each item.
Example:
for file in *.txt; do echo "Processing $file" cat $file done
This loop iterates over all .txt
files in the directory, printing the file name and its contents.
while
Loop
The while
loop continues to execute commands as long as a condition is true.
Example:
count=1 while [ $count -le 5 ]; do echo "Count: $count" count=$((count + 1)) done
This loop prints the count from 1 to 5.
10. Background Execution: Running Commands in the Background
Running commands in the background allows you to continue using the terminal while commands execute. You can achieve this by appending &
to a command.
Example:
sleep 30 &
This command delays execution for 30 seconds but runs in the background, freeing the terminal for other tasks.
To view background jobs, use the jobs
command. To bring a background job to the foreground, use fg
.
11. Using tee
for Simultaneous Output
The tee
command reads from standard input and writes to standard output and files simultaneously. It’s useful when you need to log output while also displaying it on the screen.
Example:
ls -l | tee filelist.txt
This command lists files and directories in long format, displaying the output on the screen and writing it to filelist.txt
at the same time.
Conclusion
Understanding command combinations in Linux can significantly enhance your efficiency and control over the system. By mastering logical operators, pipelines, redirection, and other techniques, you can create powerful command sequences that save time and automate tasks.
Whether you’re managing files, processing data, or scripting complex operations, these command combinations provide the tools you need to make the most out of Linux. Practice these techniques regularly, and they will become second nature, making your Linux experience more productive and enjoyable.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut