How to Create a Progress Bar in Bash
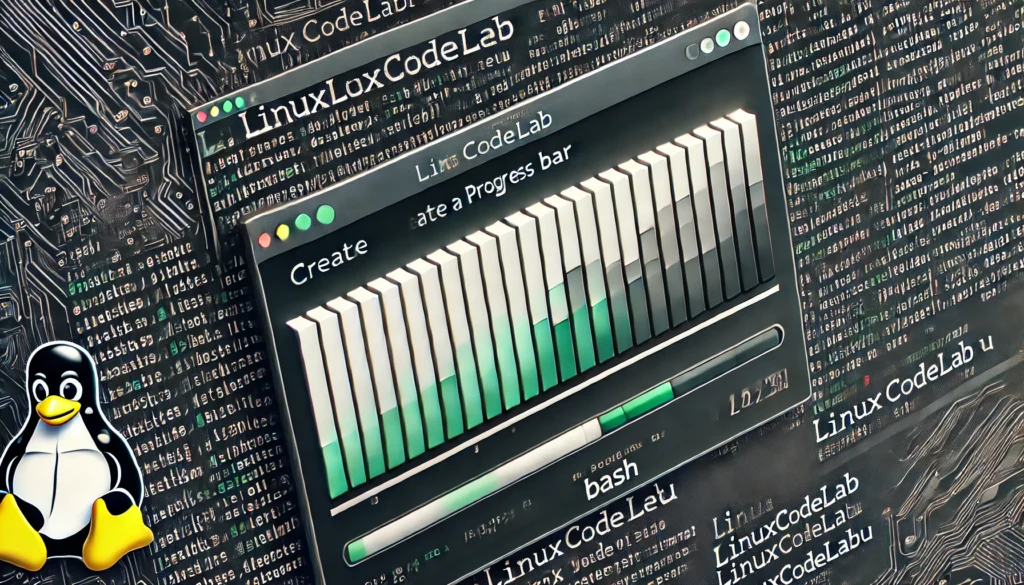
Creating a progress bar in Bash is a useful way to visualize the progress of scripts. Whether you’re copying files, downloading data, or performing complex computations, a progress bar can make the experience more interactive and user-friendly. This article will guide you through the process of creating a simple yet effective progress bar in Bash.
Understanding the Basics of Bash Scripting
Before diving into creating a progress bar, it’s essential to understand some basic concepts of Bash scripting. Bash (Bourne Again SHell) is a command processor that typically runs in a text window where the user types commands. Scripts are text files that contain a series of commands that the shell executes in sequence.
Bash scripts are used for automating tasks, and they can range from simple sequences of commands to more complex programs with loops, conditionals, and functions.
What Is a Progress Bar?
A progress bar is a graphical representation that shows the completion status of a task. It usually consists of a bar that fills up as the task progresses. In a terminal, the progress bar is displayed as a series of characters (such as “=” or “#”) that gradually fill the screen as the task moves towards completion.
Why Use a Progress Bar in Bash?
Progress bars provide visual feedback that can help users understand how long a task might take. This is particularly useful for long-running operations where users might wonder if the script is still running. By adding a progress bar, you make your scripts more user-friendly and easier to monitor.
Steps to Create a Progress Bar in Bash
Creating a progress bar in Bash involves a few simple steps. These include setting up a loop, calculating the progress, and printing the bar to the terminal.
Step 1: Set Up a Loop
The first step in creating a progress bar is setting up a loop that represents the task you’re performing. For example, if you’re simulating a task that takes 100 steps, you would create a loop that iterates 100 times.
#!/bin/bash total=100 for i in $(seq 1 $total) do # Simulate task here sleep 0.1 done
In this script, total
represents the number of steps, and sleep 0.1
simulates a delay to represent work being done.
Step 2: Calculate the Progress
Next, you need to calculate the progress. This involves determining how far along the task is at each step of the loop. You can do this by dividing the current step number by the total number of steps and multiplying by 100 to get a percentage.
progress=$((i * 100 / total))
This line of code calculates the progress as a percentage.
Step 3: Print the Progress Bar
To print the progress bar, you’ll use the calculated progress to determine how many characters to display. A typical approach is to display a bar with a fixed length, such as 50 characters. You can calculate the number of characters to display by multiplying the progress percentage by the total length of the bar and dividing by 100.
bar_length=50 filled_length=$((progress * bar_length / 100))
You then print the progress bar using a combination of filled and unfilled characters.
bar=$(printf "%-${bar_length}s" "=") echo -ne "[${bar:0:filled_length}] $progress% Completer"
The printf
command formats the bar to have a fixed length, and the echo -ne
command prints the bar on the same line, updating it as the loop progresses.
Step 4: Putting It All Together
Here’s the complete script that combines all the steps to create a working progress bar:
#!/bin/bash total=100 bar_length=50 for i in $(seq 1 $total) do progress=$((i * 100 / total)) filled_length=$((progress * bar_length / 100)) bar=$(printf "%-${bar_length}s" "=") echo -ne "[${bar:0:filled_length}] $progress% Completer" sleep 0.1 done echo
This script will display a progress bar that fills up as the loop runs, providing a visual indication of progress.
Customizing the Progress Bar
You can customize the progress bar in several ways to suit your needs. Here are a few ideas:
1. Change the Bar Character
You can change the character used to fill the bar. For example, you might prefer using #
instead of =
.
bar=$(printf "%-${bar_length}s" "#")
2. Adjust the Bar Length
If you want a longer or shorter progress bar, simply change the bar_length
variable.
bar_length=30
3. Add a Task Name
If you’re performing multiple tasks, you can display the name of the current task alongside the progress bar.
echo -ne "Task 1: [${bar:0:filled_length}] $progress% Completer"
4. Show Time Remaining
For more advanced users, you can estimate and display the time remaining based on the current progress and elapsed time. This involves recording the start time and calculating the remaining time based on the progress.
start_time=$(date +%s) for i in $(seq 1 $total) do progress=$((i * 100 / total)) filled_length=$((progress * bar_length / 100)) bar=$(printf "%-${bar_length}s" "=") elapsed_time=$(($(date +%s) - start_time)) estimated_total_time=$((elapsed_time * 100 / progress)) remaining_time=$((estimated_total_time - elapsed_time)) echo -ne "[${bar:0:filled_length}] $progress% Complete, $remaining_time seconds remainingr" sleep 0.1 done echo
This adds a rough estimate of the time remaining, making the progress bar even more informative.
Conclusion
Creating a progress bar in Bash is a simple yet powerful way to enhance your scripts. It provides visual feedback and makes long-running tasks easier to monitor. By following the steps outlined in this article, you can create a customizable progress bar that suits your needs. Whether you’re automating tasks or creating interactive scripts, adding a progress bar can significantly improve the user experience.
Final Thoughts
Remember that Bash is a versatile scripting language, and the possibilities for customization are endless. Experiment with different styles and features to make your progress bars even more effective. Happy scripting!
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut