Interact with Docker containers using docker exec
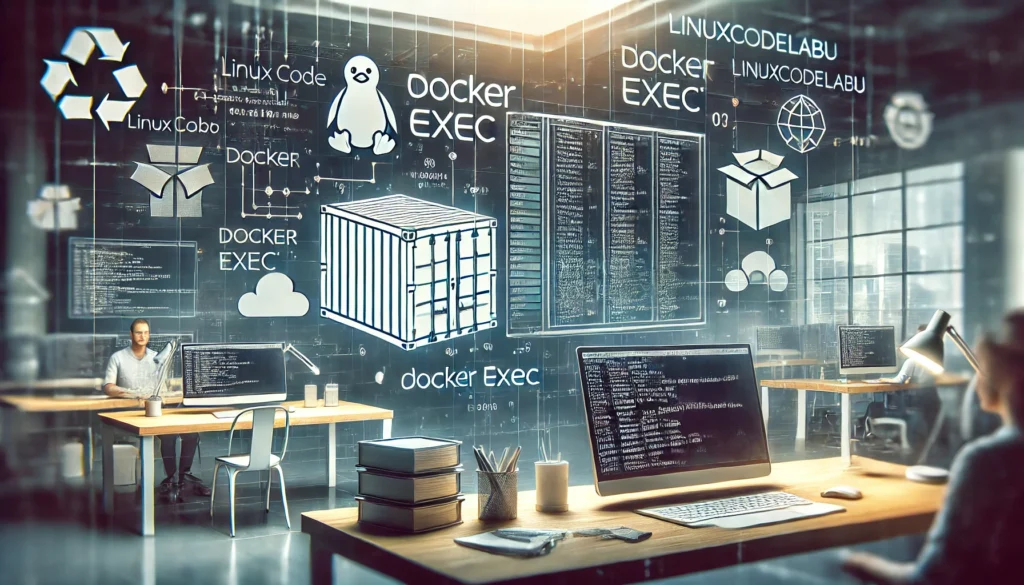
Docker has revolutionized the way we develop, deploy, and manage applications. By containerizing applications, Docker provides a lightweight, consistent, and efficient environment for both development and production. However, to fully leverage Docker’s capabilities, you need to understand how to interact with running containers. One of the most powerful commands for this purpose is docker exec
. This article will provide a detailed exploration of docker exec
, explaining what it is, how to use it effectively, and some best practices.
What Is docker exec
?
The docker exec
command allows you to run commands inside a running Docker container. This can be useful for various tasks, such as debugging, inspecting processes, or making quick changes to the environment. Unlike docker run
, which starts a new container, docker exec
lets you interact with containers that are already up and running. This capability is crucial for live troubleshooting and managing applications without restarting containers.
Why Use docker exec
?
Before diving into how to use docker exec
, it’s important to understand why you might need it. Here are some scenarios where docker exec
is particularly useful:
- Debugging and Troubleshooting: If an application in a container isn’t working as expected, you can use
docker exec
to run commands inside the container and diagnose the issue. - Monitoring and Inspecting: You can monitor resource usage, check logs, and inspect processes within the container without stopping it.
- Running Maintenance Tasks: Sometimes, you need to update configurations, install packages, or perform other maintenance tasks on a running container.
docker exec
lets you do this seamlessly. - Interactive Development: During development, you might want to test specific commands or configurations in a running container.
docker exec
allows you to do this interactively.
Basic Syntax of docker exec
The basic syntax of the docker exec
command is as follows:
docker exec [OPTIONS] CONTAINER COMMAND [ARG...]
- OPTIONS: These are flags that modify the behavior of
docker exec
. Common options include-it
for interactive mode and-u
to specify a user. - CONTAINER: This is the name or ID of the container you want to interact with.
- COMMAND: This is the command you want to run inside the container.
- ARG: These are optional arguments passed to the command.
Commonly Used Options
Understanding the options available with docker exec
can help you use the command more effectively. Here are some of the most commonly used options:
-it
: This option combines-i
(interactive) and-t
(pseudo-TTY). It allows you to run commands in an interactive shell within the container.-u
: This option lets you specify a user to run the command as, which is useful for running commands with different permissions.--env
: You can use this option to pass environment variables to the command being executed.--workdir
: This option sets the working directory inside the container before running the command.
Examples of Using docker exec
To get a practical understanding, let’s explore some examples of using docker exec
.
Running a Simple Command
Suppose you have a running container named web_server
, and you want to list the files in its /var/www
directory. You can use docker exec
as follows:
docker exec web_server ls /var/www
This command will execute ls /var/www
inside the web_server
container and display the results.
Accessing an Interactive Shell
Sometimes, you might need to access an interactive shell inside a running container. This is particularly useful for debugging or manual operations. For example, to access a bash shell inside the web_server
container, you can use:
docker exec -it web_server bash
The -it
option makes the shell interactive, allowing you to execute further commands directly within the container.
Running Commands as a Different User
If you need to run a command as a different user, you can use the -u
option. For instance, to list the contents of the /home
directory as the user admin
, use:
docker exec -u admin web_server ls /home
This command will run ls /home
as the admin
user inside the container.
Passing Environment Variables
You can pass environment variables to the command inside the container using the --env
option. For example, to set a variable MY_VAR
to 123
and echo it within the container, use:
docker exec --env MY_VAR=123 web_server bash -c 'echo $MY_VAR'
This command will output 123
, demonstrating that the environment variable was successfully passed to the container.
Best Practices for Using docker exec
While docker exec
is a powerful tool, it’s important to follow best practices to avoid potential issues.
Use Interactive Mode with Caution
Interactive mode (-it
) is extremely useful but can be dangerous if used carelessly. Always ensure that commands run in interactive mode are necessary and understand their potential impact on the running container.
Monitor Resource Usage
Using docker exec
to run resource-intensive commands can affect the performance of your containerized application. Be mindful of the resources used by commands executed inside containers, especially in production environments.
Clean Up After Execution
Commands run via docker exec
can leave behind temporary files, processes, or changes that might impact the container’s state. It’s good practice to clean up after executing commands to maintain the container’s integrity.
Avoid Frequent Use in Production
While docker exec
is indispensable for debugging and ad-hoc tasks, avoid relying on it too frequently in production. Instead, automate routine maintenance tasks and configurations through Dockerfiles or orchestrators like Kubernetes.
Advanced Use Cases
Beyond the basics, docker exec
can be used in more advanced scenarios to enhance your container management.
Executing Commands in Multiple Containers
If you need to run a command across multiple containers, you can script the process using docker exec
. For example, using a loop in a bash script:
for container in $(docker ps -q); do docker exec $container <command> done
This script runs the specified command inside each running container.
Combining with Other Docker Commands
docker exec
can be combined with other Docker commands to perform complex operations. For instance, you can inspect a container’s state before executing a command:
if docker inspect -f '{{.State.Running}}' web_server; then docker exec web_server <command> fi
This ensures the command is only executed if the container is running.
Common Pitfalls and How to Avoid Them
Despite its utility, docker exec
can lead to pitfalls if not used correctly.
Modifying Container State
Running commands that modify the container’s state can lead to unexpected results, especially if those changes aren’t persistent. For example, installing new software or altering configurations via docker exec
might not survive a container restart.
Solution: Make necessary changes in a Dockerfile and rebuild the container image to ensure consistency.
Security Risks
Using docker exec
to run commands with elevated privileges (e.g., as root) can introduce security risks, especially if unauthorized users gain access.
Solution: Restrict access to Docker and avoid running containers as root whenever possible. Always use the -u
option to specify non-privileged users for commands.
Performance Impact
Running resource-intensive tasks via docker exec
can degrade container performance, affecting the application’s overall stability.
Solution: Monitor resource usage and prefer offloading heavy tasks to dedicated environments outside of Docker.
Conclusion
The docker exec
command is an invaluable tool for interacting with running Docker containers. Whether you need to debug, inspect, or perform maintenance, docker exec
provides a direct and efficient way to manage containers without interrupting their operation. By following best practices and being aware of potential pitfalls, you can use docker exec
to maintain and troubleshoot containers effectively.
As you continue to work with Docker, mastering docker exec
will undoubtedly enhance your container management skills, enabling you to respond quickly to issues and maintain the smooth operation of your containerized applications.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut