Downloading YouTube Videos as MP3 Files
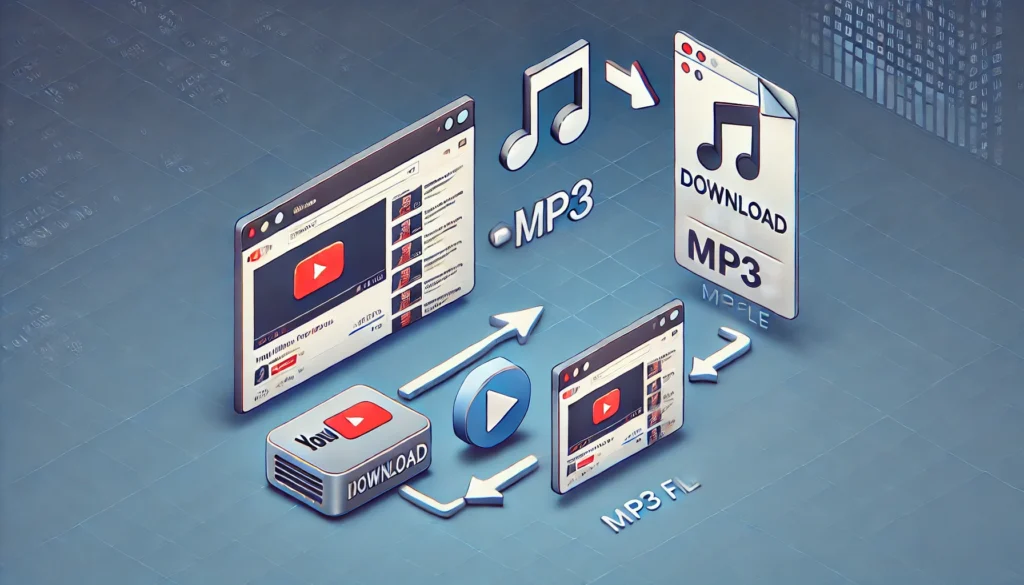
Downloading audio files from YouTube is a task that many users find necessary, whether for offline listening, creating soundtracks, or various other reasons. Python, with its rich ecosystem of libraries and frameworks, provides a straightforward way to achieve this task using the pytube
library and tkinter
for the graphical user interface (GUI). This article walks you through a Python script that enables users to download MP3 files from YouTube easily.
Script Overview and Translation
The script is written in Python and uses the following key components:
- Tkinter: A standard GUI library in Python for creating windows, dialogs, and user input forms.
- Pytube: A Python library for downloading YouTube videos. It provides methods to access streams and handle various formats.
Translation of the Script
Here’s the English translation of the Bulgarian script you provided:
import tkinter as tk
from tkinter import filedialog
from tkinter import messagebox
from pytube import YouTube
import os
destination_variable = None
def download_mp3(url, destination='.', quality='best'):
global destination_variable
try:
yt = YouTube(url)
audio_stream = yt.streams.filter(only_audio=True).first()
destination_folder = destination_variable.get() if isinstance(destination_variable, tk.StringVar) else destination_variable
if audio_stream:
downloaded_file = audio_stream.download(output_path=destination_folder)
base, ext = os.path.splitext(downloaded_file)
new_file = base + '.mp3'
os.rename(downloaded_file, new_file)
messagebox.showinfo("Download Complete", "The file was successfully downloaded and converted to MP3 format.")
return new_file
else:
messagebox.showerror("Error", "No audio stream was found for this URL.")
return None
except Exception as e:
messagebox.showerror("Error", f"An error occurred during download: {str(e)}")
return None
def choose_destination():
global destination_variable
chosen_folder = filedialog.askdirectory()
destination_variable.set(chosen_folder)
def paste_url(root):
clipboard_text = root.clipboard_get()
url_entry.delete(0, tk.END)
url_entry.insert(0, clipboard_text)
def main():
global destination_variable
global url_entry
root = tk.Tk()
root.title("MP3 File Downloader")
root.geometry("500x250")
url_label = tk.Label(root, text="URL Address:")
url_label.pack()
url_entry = tk.Entry(root, width=50)
url_entry.pack()
paste_button = tk.Button(root, text="Paste URL", command=lambda: paste_url(root))
paste_button.pack()
destination_label = tk.Label(root, text="Destination:")
destination_label.pack()
destination_variable = tk.StringVar(root, value=os.getcwd())
destination_entry = tk.Entry(root, textvariable=destination_variable)
destination_entry.pack()
destination_button = tk.Button(root, text="Choose Folder", command=choose_destination)
destination_button.pack()
quality_label = tk.Label(root, text="Choose Audio Quality:")
quality_label.pack()
quality_options = ['best', 'high', 'medium', 'low']
quality_variable = tk.StringVar(root)
quality_variable.set(quality_options[0])
quality_menu = tk.OptionMenu(root, quality_variable, *quality_options)
quality_menu.pack()
download_button = tk.Button(root, text="Download", command=lambda: download_mp3(url_entry.get(), destination_variable, quality_variable.get()))
download_button.pack()
root.mainloop()
if __name__ == "__main__":
main()
Explanation of the Script
Importing Necessary Libraries
The script begins by importing the required libraries:
import tkinter as tk
from tkinter import filedialog, messagebox
from pytube import YouTube
import os
tkinter
: Used for creating the GUI. It includes components likefiledialog
for directory selection andmessagebox
for user notifications.pytube
: Used for interacting with YouTube and downloading video/audio streams.os
: Used for file and directory operations.
Setting Up the Download Function
The core function of this script is download_mp3
, which handles the downloading of the audio stream and its conversion to MP3 format:
def download_mp3(url, destination='.', quality='best'):
...
This function accepts three parameters:
url
: The YouTube video URL.destination
: The folder where the downloaded file will be saved.quality
: The desired audio quality (not fully utilized in this script but can be expanded).
Interacting with YouTube
Within the download_mp3
function, the YouTube
object is created using the provided URL:
yt = YouTube(url)
The script then filters the available streams to select only the audio streams:
audio_stream = yt.streams.filter(only_audio=True).first()
If an audio stream is found, the script proceeds to download and rename the file to an MP3 format:
downloaded_file = audio_stream.download(output_path=destination_folder)
base, ext = os.path.splitext(downloaded_file)
new_file = base + '.mp3'
os.rename(downloaded_file, new_file)
The user is notified upon completion or if an error occurs.
User Interface Components
The GUI is constructed using tkinter
. The main window is set up and populated with various widgets:
- URL Entry and Label: For inputting the YouTube URL.
- Paste Button: To paste a URL from the clipboard.
- Destination Selection: Allows the user to choose a download destination.
- Quality Selection: Provides options for selecting the audio quality.
- Download Button: Initiates the download process.
Running the Application
The main
function initializes the Tkinter root window and configures it with labels, buttons, and entry fields. It then starts the event loop with root.mainloop()
.
Conclusion
This script provides a simple, user-friendly way to download YouTube videos as MP3 files using Python. It effectively leverages the pytube
library for accessing YouTube’s streams and tkinter
for creating an intuitive GUI. The step-by-step process includes URL input, destination selection, and audio quality selection, making it a practical tool for users looking to save audio content from YouTube.
Adding Visuals
To enhance understanding, visuals can be added, such as screenshots of the application interface and diagrams explaining the flow of the script. Here is an example of what the application window might look like:
Main Application Window
Figure 1: The main interface where users can enter a YouTube URL, select a destination folder, and choose the audio quality.
Folder Selection Dialog
Figure 2: The folder selection dialog that allows users to choose where to save the downloaded MP3 file.
Future Enhancements
While the current script is functional, there are several potential improvements:
- Support for Different Audio Formats: Adding the option to download files in formats other than MP3.
- Audio Quality Control: Implementing actual audio quality selection by filtering streams based on bitrate.
- Progress Bar: Adding a progress bar to provide visual feedback during the download process.
This script serves as a foundation for more advanced applications and is a practical introduction to GUI programming with tkinter
and web scraping with pytube
.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut