JavaScript content protection: Copy restriction example in WordPress
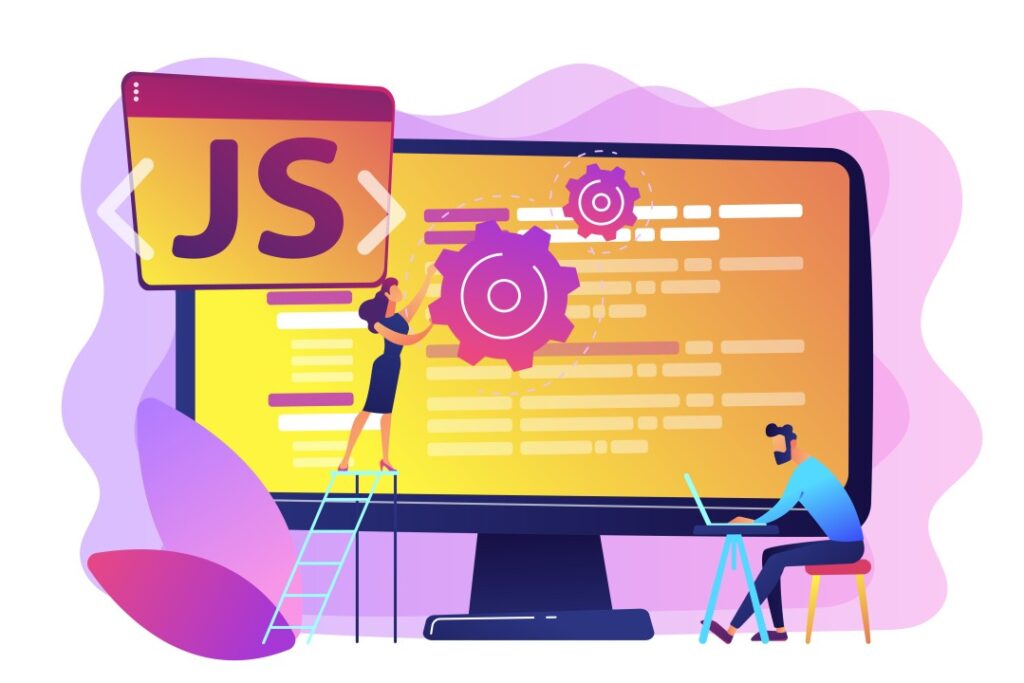
In today’s digital age, protecting your website’s content is essential. Unauthorized copying can lead to copyright infringement and reduce your content’s uniqueness in search engines. One effective way to protect your content is by using JavaScript to limit copying. This article explains how to create a simple JavaScript script to restrict text copying on your website, focusing on WordPress integration.
Why protect your content?
Website content is a valuable asset that reflects your brand, ideas, and expertise. When someone copies your content without permission, it can have several negative consequences:
- Loss of original content: Search engines prioritize original content. If your content is copied and reposted, it can dilute its uniqueness and impact your search rankings.
- Violation of copyright: Unauthorized copying can lead to copyright infringement, which is illegal and can harm your reputation.
- Decreased value: If your content is freely copied, it loses its value as an exclusive resource for your audience.
To mitigate these risks, you can use JavaScript to implement content protection measures, particularly on WordPress websites.
Step-by-step guide to implementing content protection
Step 1: Adding JavaScript to WordPress
The first step is to add custom JavaScript to your WordPress site. This will allow you to control how your content behaves when someone tries to copy it.
Using the functions.php
file
You can add JavaScript directly through your theme’s functions.php
file. This method is effective and ensures the script is included globally on your site.
Here’s a simple code snippet to add in functions.php
:
function add_admin_check_script() {
// Check if the user is logged in and is an administrator
$is_admin = current_user_can('administrator') ? 'true' : 'false';
?>
<script type="text/javascript">
// Define a global variable isUserAdmin
window.isUserAdmin = <?php echo $is_admin; ?>;
</script>
<?php
}
add_action('wp_head', 'add_admin_check_script');
This code checks if the current user is an administrator and then injects a JavaScript variable, isUserAdmin
, with a value of true
or false
.
Why use the functions.php
file?
Adding JavaScript through functions.php
ensures that your script is loaded on every page of your site. This approach also allows you to make use of WordPress’s capabilities to identify user roles.
Step 2: Adding the JavaScript for copy restriction
Once you’ve defined whether the user is an admin, the next step is to implement the JavaScript that controls the copying behavior.
Here’s the script to add:
<script type="text/javascript">
// Function to check if the user is an administrator
function isAdmin() {
return window.isUserAdmin === 'true';
}
// Event listener for copy actions
document.addEventListener('copy', function(e) {
// Allow copying for admins or if the text length is 250 characters or less
if (isAdmin() || window.getSelection().toString().length <= 250) {
return; // Do nothing, allow the copy
}
// Prevent default copy action and replace copied text
e.preventDefault();
var yourText = 'Content copying is restricted on this site.';
e.clipboardData.setData('text/plain', yourText);
});
</script>
This script works as follows:
- Check user role: It uses the
isAdmin
function to check if the user is an administrator. - Monitor copy action: It listens for the
copy
event on the document. - Restrict copying: If the user is not an admin and the selected text is more than 250 characters, it prevents the default copy action and replaces the clipboard content with a custom message.
Step 3: Testing and troubleshooting
After implementing the script, it’s important to test it to ensure it works as expected across different scenarios.
Testing on various roles
- Admin users: Ensure that administrators can copy text without restrictions.
- Regular users: For non-admin users, verify that copying is restricted and the custom message appears.
Cross-browser testing
Make sure to test the script on various browsers (e.g., Chrome, Firefox, Safari) and devices (e.g., mobile, tablet) to ensure compatibility.
How the script works
Let’s break down the functionality of the script:
Checking the user’s role
The script first checks if the user is an admin by accessing the global variable isUserAdmin
. This is set to true
if the user is an admin and false
otherwise. This check is crucial because admins should have full control over the content.
Limiting copying based on text length
The script also checks if the selected text is 250 characters or less. If it is, the script allows copying. This length can be adjusted depending on your needs, but it’s a useful way to allow small excerpts to be copied, which can be beneficial for users who want to share short snippets.
Preventing the default copy action
If the user is not an admin and the selected text is more than 250 characters, the script prevents the default copy action using e.preventDefault()
. This stops the standard behavior of copying the selected text to the clipboard.
Replacing copied content with a custom message
Instead of the selected text, a custom message is copied to the clipboard. This message can be personalized to suit your website’s tone and branding.
Conclusion
By implementing this simple JavaScript script, you can add an extra layer of protection to your WordPress site’s content. It’s important to note, however, that this is not a foolproof method of content protection. Savvy users can still find ways to bypass the script, such as by disabling JavaScript in their browser.
Additional considerations
- Legal protection: In addition to technical measures, consider legal avenues such as copyright notices and legal action against infringers.
- Content value: Remember that the goal is to protect your content’s value. Ensure that your protection measures do not interfere with user experience, particularly for legitimate users who may need to copy small excerpts.
Frequently asked questions (FAQs)
Can this script be bypassed?
Yes, technically skilled users can bypass this script. This solution is intended as a deterrent and a first line of defense.
Why limit copying to 250 characters?
The 250-character limit is just an example. It allows users to copy short excerpts, which can be useful for quoting or sharing snippets.
How can I change the custom message?
You can change the message by modifying the yourText
variable in the JavaScript code to whatever text you prefer.
Will this script work on all browsers?
The script is compatible with most modern browsers. However, always test it across different browsers and devices to ensure consistency.
Can I implement this script without modifying functions.php
?
Yes, you can add this script through WordPress plugins or directly in your theme’s JavaScript files. Using functions.php
is just one method that integrates well with WordPress’s structure.
By following this guide, you can help protect your content from unauthorized copying while maintaining control over your website’s user experience.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut