OpenResty: Everything you need to know
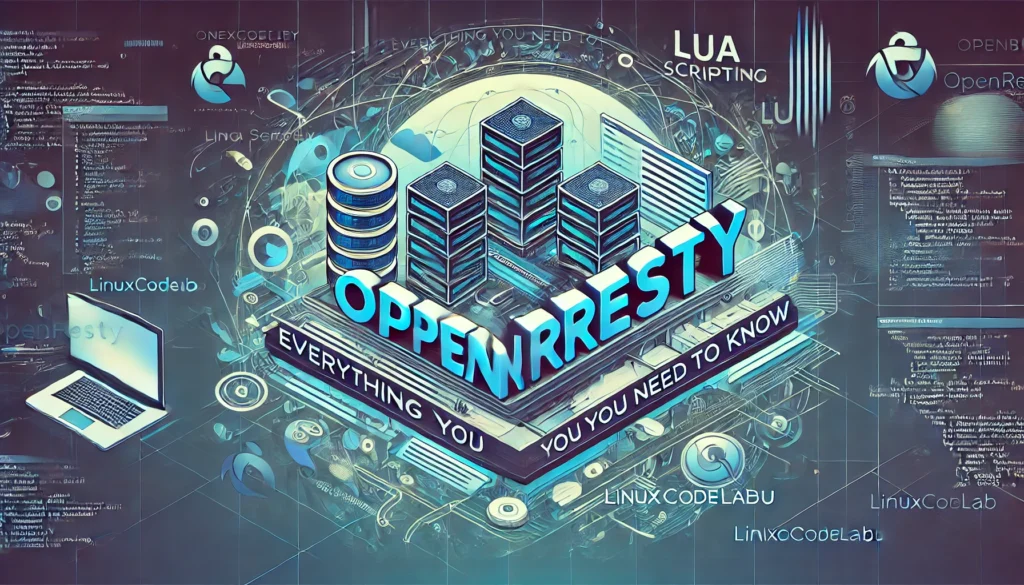
OpenResty is an advanced web platform that extends Nginx, a popular open-source web server, to provide a powerful, high-performance web framework. By integrating Lua scripting language with Nginx’s core, OpenResty enables developers to build dynamic web applications, APIs, and services efficiently. In this article, we will explore OpenResty in detail, covering its architecture, key features, common use cases, installation, and practical examples.
Introduction to OpenResty
OpenResty is designed to handle HTTP traffic and build scalable web applications by leveraging Nginx and LuaJIT. It is widely used in high-traffic environments due to its efficiency and flexibility. While Nginx is known for its speed and low memory footprint, OpenResty enhances these capabilities by embedding the Lua language, allowing developers to execute Lua code directly within the Nginx server.
Key Features of OpenResty
- Embedded Lua Scripting: OpenResty allows developers to write and execute Lua scripts within Nginx, providing the flexibility to handle complex web tasks.
- Non-blocking I/O: The platform supports non-blocking I/O operations, making it suitable for handling a large number of concurrent connections efficiently.
- High Performance: Built on the fast and lightweight Nginx server, OpenResty inherits its high performance and ability to handle high traffic with low latency.
- Extensibility: OpenResty comes with a wide range of built-in libraries and modules, making it easy to extend and customize for specific needs.
- Compatibility: It is compatible with many Nginx modules, allowing developers to use existing Nginx features alongside Lua scripting.
- Asynchronous Processing: OpenResty supports asynchronous processing, enabling efficient handling of I/O-bound tasks and improving overall system throughput.
Architecture of OpenResty
OpenResty’s architecture is built on top of Nginx and LuaJIT. The core components include:
- Nginx: The foundation of OpenResty, Nginx serves as the HTTP server and reverse proxy. It handles client requests and manages connections efficiently.
- LuaJIT: A Just-In-Time (JIT) compiler for Lua, LuaJIT enhances the performance of Lua scripts by compiling them into machine code at runtime.
- Modules and Libraries: OpenResty includes a variety of modules and libraries, such as
ngx_lua
,lua-resty-core
, andlua-resty-redis
, that provide additional functionality for web development. - Lua Code Execution: Lua scripts are embedded within Nginx configuration files and executed during request processing. This allows for dynamic content generation and advanced request handling.
Common Use Cases for OpenResty
OpenResty is a versatile platform used in various scenarios, including:
- API Gateway: OpenResty can be used as an API gateway to route, manage, and secure API requests. It provides features like rate limiting, authentication, and load balancing.
- Dynamic Web Applications: By embedding Lua scripts, developers can create dynamic web applications with custom logic, session management, and real-time updates.
- Reverse Proxy: OpenResty can act as a reverse proxy, directing incoming requests to different backend servers based on routing rules defined in Lua.
- Content Delivery Networks (CDNs): With its high performance and low latency, OpenResty is suitable for building CDNs that cache and deliver content efficiently.
- Web Application Firewall (WAF): OpenResty can be configured to act as a WAF, inspecting and filtering incoming HTTP requests to protect against security threats.
- Microservices Architecture: OpenResty is often used in microservices architectures to manage communication between services, handle service discovery, and implement circuit breakers.
Installation and Setup
Setting up OpenResty is straightforward, but it requires a few steps to ensure a proper installation. Below is a basic guide to installing OpenResty on a Linux system.
Prerequisites
Before installing OpenResty, ensure that your system meets the following requirements:
- A Linux-based operating system (e.g., Ubuntu, CentOS)
- Root or sudo access to install packages
- Basic knowledge of command-line operations
Installation Steps
- Install Dependencies: Start by installing the necessary dependencies, such as
wget
,gcc
,pcre
,zlib
, andopenssl
.bashCopy codesudo apt-get update sudo apt-get install -y wget build-essential libpcre3 libpcre3-dev zlib1g zlib1g-dev libssl-dev
- Download OpenResty: Download the latest version of OpenResty from the official website or using the command line.bashCopy code
wget https://openresty.org/download/openresty-x.x.x.tar.gz tar -zxvf openresty-x.x.x.tar.gz cd openresty-x.x.x
- Configure and Compile: Configure the build options and compile OpenResty.bashCopy code
./configure --with-http_ssl_module --with-pcre make sudo make install
- Verify Installation: After installation, verify that OpenResty is installed correctly by checking the version.bashCopy code
/usr/local/openresty/nginx/sbin/nginx -v
Basic Configuration
Once installed, you can configure OpenResty by editing the Nginx configuration file, typically located at /usr/local/openresty/nginx/conf/nginx.conf
. Here is a basic example of how to embed Lua code:
http { server { listen 80; server_name example.com; location /lua { content_by_lua_block { ngx.say("Hello, OpenResty!") } } } }br>
In this example, a simple Lua script responds with “Hello, OpenResty!” when a request is made to /lua
.
Practical Examples
Example 1: Simple Reverse Proxy
One of the common uses of OpenResty is as a reverse proxy. Below is an example of how to set up a basic reverse proxy using OpenResty.
http { server { listen 80; server_name proxy.example.com; location / { proxy_pass http://backend.example.com; } } }br>
In this configuration, requests to proxy.example.com
are forwarded to backend.example.com
.
Example 2: Rate Limiting API Requests
OpenResty can be used to implement rate limiting for API requests, ensuring that clients do not exceed a predefined request limit.
http { lua_shared_dict my_limit_store 10m; server { listen 80; server_name api.example.com; location / { access_by_lua_block { local limit_req = require "resty.limit.req" local lim, err = limit_req.new("my_limit_store", 200, 100) if not lim then ngx.log(ngx.ERR, "failed to instantiate a resty.limit.req object: ", err) return ngx.exit(500) end local key = ngx.var.binary_remote_addr local delay, err = lim:incoming(key, true) if not delay then if err == "rejected" then return ngx.exit(503) end ngx.log(ngx.ERR, "failed to limit req: ", err) return ngx.exit(500) end if delay >= 0.001 then ngx.sleep(delay) end } proxy_pass http://backend.example.com; } } }br>
This example demonstrates how to limit the number of requests per second to an API endpoint, protecting the backend from being overwhelmed by too many requests.
Example 3: Custom Authentication
OpenResty can also be used to implement custom authentication mechanisms using Lua.
http { server { listen 80; server_name auth.example.com; location / { access_by_lua_block { local auth_header = ngx.req.get_headers()["Authorization"] if auth_header ~= "Bearer your-token" then return ngx.exit(401) end } proxy_pass http://backend.example.com; } } }br>
In this configuration, requests are authenticated by checking the Authorization
header against a predefined token before being forwarded to the backend.
Performance Optimization and Tuning
OpenResty, while efficient, requires proper configuration and tuning to achieve optimal performance. Here are some best practices:
- Use LuaJIT: Ensure that LuaJIT is enabled to benefit from JIT compilation, which significantly improves the performance of Lua scripts.
- Optimize Nginx Configuration: Tuning Nginx worker processes, buffer sizes, and timeouts can enhance the overall performance of OpenResty.
- Leverage Caching: Use OpenResty’s caching capabilities to store frequently requested data in memory, reducing the load on backend services.
- Monitor Performance: Regularly monitor the performance of your OpenResty setup using tools like
nginx-vts-module
or custom Lua scripts to track metrics. - Asynchronous I/O: Utilize non-blocking I/O operations to handle large numbers of concurrent connections efficiently.
Conclusion
OpenResty is a powerful and flexible web platform that extends the capabilities of Nginx by embedding Lua scripting. Its high performance, non-blocking I/O, and extensive feature set make it suitable for building scalable web applications, API gateways, and microservices.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut