Monitoring Docker Containers with a Bash Script
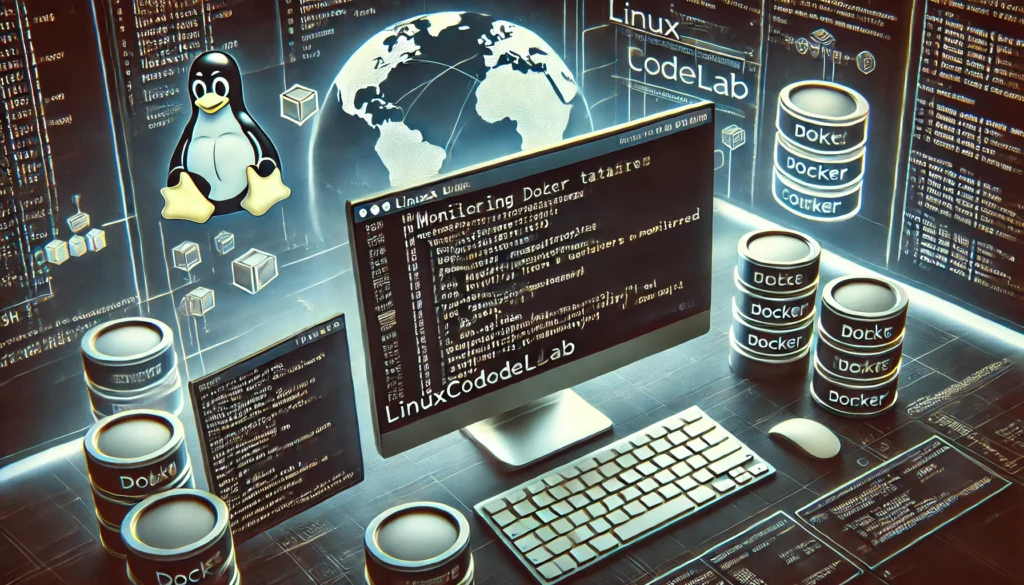
Monitoring Docker Containers with a Bash Script: A Step-by-Step Guide
Managing Docker containers efficiently is crucial for any system administrator. Docker is widely used for containerization, but monitoring the status of these containers can be challenging. This article introduces a simple Bash script to check the status of Docker containers and send notifications via Telegram if any containers are not running.
What the Script Does
The script performs several tasks:
- Lists all Docker containers: It retrieves the names of all containers on the system.
- Checks container status: It checks if each container is running or stopped.
- Sends Telegram notifications: If any container is stopped, the script sends an alert via Telegram.
- Maintains a status file: The script maintains a status file to track changes and avoid sending redundant notifications.
This script is designed for administrators who need to monitor Docker containers proactively. By automating the monitoring process, it helps ensure that any issues with containers are promptly addressed.
Setting Up the Script
Before diving into the script, you need a few prerequisites:
- Docker: Ensure Docker is installed and running on your system.
- Telegram Bot: Set up a Telegram bot and obtain the bot token and chat ID.
- Bash: The script is written in Bash, so a Unix-like environment is needed.
#!/bin/bash
TOKEN="" # Insert your Telegram bot token here
chat_id="" # Insert your chat ID here
FILE="container-check"
docker_list=$(docker ps -a --format "{{.Names}}")
stopped_containers=""
for container in $docker_list; do
status=$(docker container inspect -f '{{.State.Status}}' "$container")
if [[ "$status" != "running" ]]; then
container_name="${container//_/-}"
stopped_containers+="$container_name\n"
fi
done
if [[ -n "$stopped_containers" ]]; then
echo 0 > "$FILE"
else
echo 1 > "$FILE"
fi
status_code=$(cat "$FILE")
if [[ "$status_code" -eq 1 ]]; then
if [[ -f "${FILE}.txt" ]]; then
message="🟢 *Great! All containers are working!*%0A%0A"
curl -s -X POST "https://api.telegram.org/bot${TOKEN}/sendMessage" -d chat_id="${chat_id}" -d text="${message}" -d parse_mode=Markdown
rm -f "${FILE}.txt"
fi
else
if [[ ! -f "${FILE}.txt" ]]; then
message="🔴 *Stopped containers:*%0A%0A"
message+=$(echo -e "$stopped_containers")
curl -s -X POST "https://api.telegram.org/bot${TOKEN}/sendMessage" -d chat_id="${chat_id}" -d text="${message}" -d parse_mode=Markdown
echo -e "$stopped_containers" > "${FILE}.txt"
fi
fi
- If all containers are running, the script sends a “Green” notification.
- If any container is stopped, it sends a “Red” alert with the names of the stopped containers.
- The script uses
curl
to send a message to the specified Telegram chat. - It only sends notifications when there is a change in the status of the containers, to avoid unnecessary alerts.
How to Use the Script
- Insert Your Details: Replace the
TOKEN
andchat_id
with your Telegram bot’s token and chat ID. - Make the Script Executable: Run
chmod +x script.sh
to make the script executable. - Run the Script: Execute the script manually or set it up as a cron job for automated monitoring.
Benefits of Using This Script
- Automated Monitoring: The script continuously monitors your Docker containers, reducing the need for manual checks.
- Timely Notifications: It sends alerts in real-time via Telegram, allowing you to respond quickly to any issues.
- Easy to Customize: The script is straightforward and can be easily modified to suit your specific needs.
Conclusion
Monitoring Docker containers is essential for maintaining a stable and secure environment. This simple Bash script provides an efficient way to track container status and receive timely alerts via Telegram. By automating this process, you can ensure that your containers are always up and running, with minimal manual intervention required.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut