cURL Mastery: From Beginner to Expert
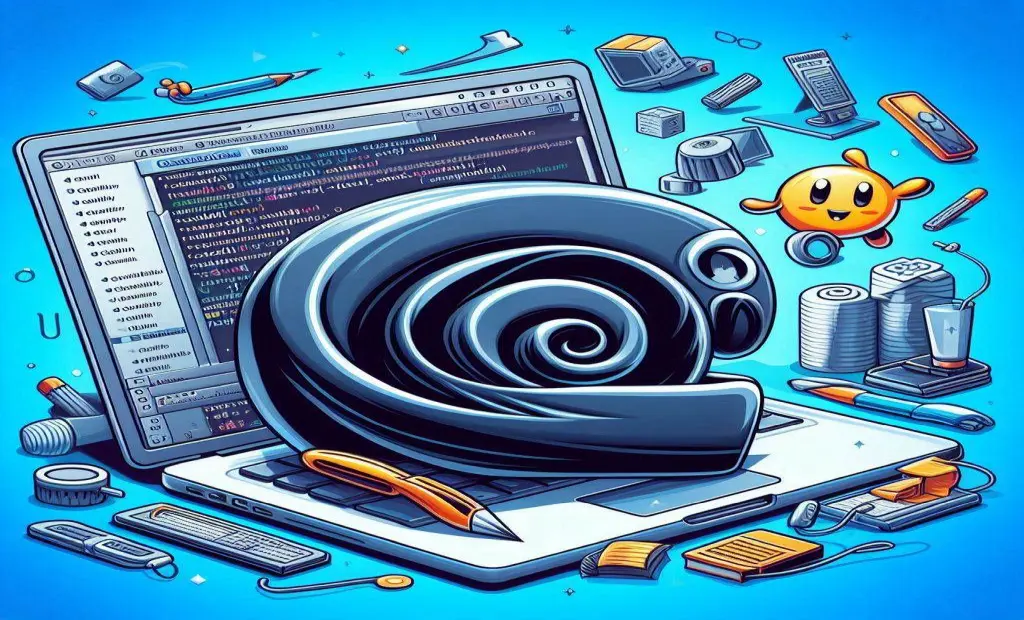
cURL Mastery: From Beginner to Expert
cURL, short for “Client URL,” is a command-line tool used to transfer data. It supports various protocols, including HTTP, HTTPS, FTP, and more. This article will guide you from the basics to advanced usage of cURL, ensuring you become proficient in its application.
Introduction to cURL
cURL is a versatile tool for interacting with web servers. It allows you to send and receive data using different protocols. Developers and system administrators use cURL for testing APIs, downloading files, and automating tasks.
Installing cURL
Before using cURL, you need to install it. The installation process varies depending on your operating system.
- Linux: Use the command
sudo apt-get install curl
. - macOS: Use Homebrew with the command
brew install curl
. - Windows: Download the appropriate version from the official cURL website. Extract the files and add the path to your system’s environment variables.
Basic Syntax of cURL
The basic syntax of a cURL command is straightforward:
curl [options] [URL]
Here, options
specify the action to be performed, and URL
is the target address.
Making Simple Requests
To make a simple GET request, use:
curl http://example.com
This command fetches the HTML content of the specified URL.
Downloading Files
To download a file, use the -o
option followed by the desired filename:
curl -o example.html http://example.com
This command saves the content of the URL to a file named example.html
.
Handling Different Protocols
cURL supports various protocols. For example, to download a file via FTP, use:
curl ftp://example.com/file.txt -o file.txt
This command downloads file.txt
from the FTP server.
Using HTTP Methods
cURL allows you to specify HTTP methods such as GET, POST, PUT, and DELETE. To send a POST request, use:
curl -X POST -d "param1=value1¶m2=value2" http://example.com
This command sends data to the server using the POST method.
Handling Authentication
Many web services require authentication. cURL supports basic authentication with the -u
option:
curl -u username:password http://example.com
This command includes the specified username and password in the request.
Working with Headers
You can include custom headers in your requests using the -H
option:
curl -H "Content-Type: application/json" http://example.com
This command adds a Content-Type
header to the request.
Saving and Loading Cookies
cURL can handle cookies, which are often used for maintaining sessions. To save cookies, use:
curl -c cookies.txt http://example.com
To load cookies from a file, use:
curl -b cookies.txt http://example.com
Uploading Files
To upload a file, use the -F
option:
curl -F "file=@path/to/file" http://example.com/upload
This command uploads the specified file to the server.
Handling Redirects
By default, cURL does not follow redirects. To enable this, use the -L
option:
curl -L http://example.com
This command follows any redirects encountered during the request.
Debugging with cURL
cURL provides options for debugging and troubleshooting. The -v
option enables verbose mode, displaying detailed information about the request and response:
curl -v http://example.com
The -I
option fetches only the headers of the response:
curl -I http://example.com
Advanced Usage
As you become more comfortable with cURL, you can explore advanced features. For example, you can use cURL in scripts to automate tasks. Here is a simple example of a bash script using cURL:
#!/bin/bash
URL="http://example.com"
RESPONSE=$(curl -s -o /dev/null -w "%{http_code}" $URL)
if [ $RESPONSE -eq 200 ]; then
echo "The request was successful."
else
echo "The request failed with status code $RESPONSE."
fi
This script checks the HTTP status code of a request and prints a message based on the result.
Conclusion
Mastering cURL involves understanding its basic syntax and exploring its many options. From making simple requests to handling authentication and debugging, cURL is a powerful tool for web interactions. By practicing and experimenting with different commands, you can become proficient in using cURL for various tasks.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut