Docker Compose: A Comprehensive Guide
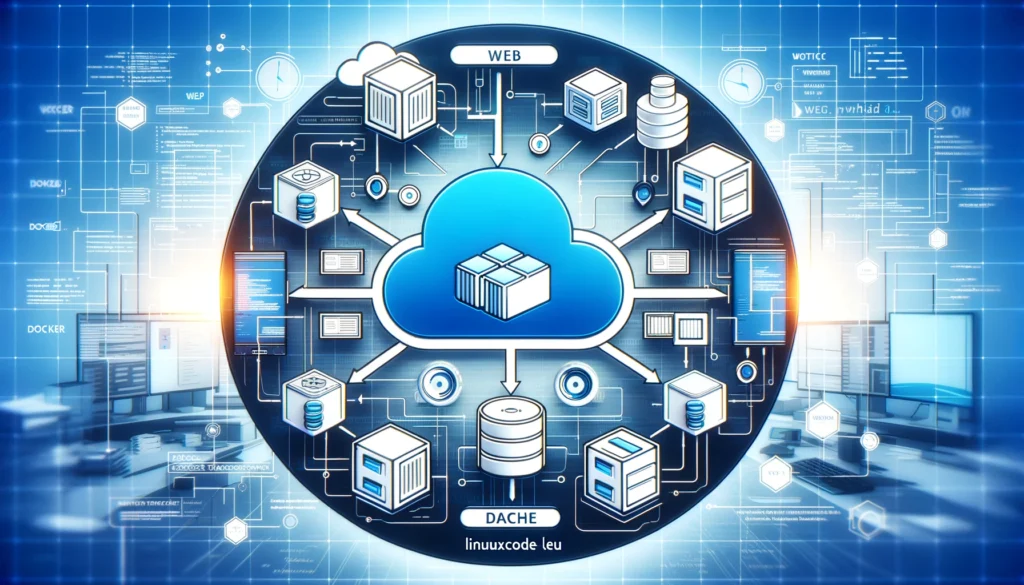
Docker Compose is a powerful tool that simplifies managing multi-container Docker applications. It’s designed to make orchestrating containers easier by allowing you to define, configure, and run multiple containers using a single YAML file. Whether you’re a developer, system administrator, or someone interested in containerization, Docker Compose can greatly enhance your workflow. This guide will take you through the essentials of Docker Compose, from understanding its basics to advanced usage.
What Is Docker Compose?
Docker Compose is an open-source tool that allows you to define and manage multi-container Docker applications. Instead of manually running multiple docker run
commands, Docker Compose enables you to describe your application’s architecture in a simple YAML file. This file, typically named docker-compose.yml
, specifies the services, networks, and volumes required by your application.
With a single command, you can create and start all the services defined in your YAML file. This approach not only saves time but also ensures consistency across different environments, such as development, testing, and production.
Why Use Docker Compose?
Docker Compose is particularly useful for managing complex applications. For instance, imagine you’re developing a web application with a frontend, backend, and database. Without Docker Compose, you’d need to manually start each service, link them, and manage their dependencies. Docker Compose simplifies this process by allowing you to manage everything from a single configuration file.
Here are some key benefits:
- Simplified Configuration: Define all services and configurations in a single file.
- Consistency: Ensure that your application runs the same way in every environment.
- Scalability: Easily scale your services by adjusting the configuration file.
- Portability: Share your Docker Compose file with others, enabling them to replicate your environment effortlessly.
- Dependency Management: Docker Compose handles the start-up order of services, ensuring dependencies are ready before your application starts.
Setting Up Docker Compose
Before using Docker Compose, ensure that Docker is installed on your machine. Docker Compose comes bundled with Docker Desktop on Windows and macOS. On Linux, you may need to install Docker Compose separately.
Step 1: Install Docker
For most platforms, Docker Desktop includes Docker Compose. If you haven’t installed Docker yet, visit the official Docker website and follow the installation instructions for your operating system.
Step 2: Verify the Installation
To check if Docker Compose is installed correctly, open a terminal and run:
docker-compose --version
This command should display the installed version of Docker Compose. If it doesn’t, double-check your installation process.
Writing a Docker Compose File
A Docker Compose file, typically named docker-compose.yml
, is written in YAML format. This file defines the services, networks, and volumes your application needs.
Let’s walk through a basic example of a docker-compose.yml
file for a simple web application using Python and Redis.
yamlversion: '3' services: web: image: python:3.8 volumes: - .:/code ports: - "5000:5000" command: python app.py redis: image: "redis:alpine"
In this example:
- version: Specifies the Docker Compose file format version.
- services: Defines the services in your application, each with its own configuration.
- web: The web service uses the Python 3.8 image and binds the host’s current directory to
/code
. It also maps port 5000 on the host to port 5000 in the container. - redis: The Redis service uses the
redis:alpine
image.
Understanding the Docker Compose File
Let’s break down the components of a Docker Compose file.
Version
The version
key specifies the version of the Docker Compose file format. Different versions support different features. Version 3 is the most commonly used and is compatible with most Docker deployments.
Services
The services
key is the heart of the Docker Compose file. It defines each container that will run as part of your application. Each service can specify the following:
- image: The Docker image to use for the service.
- build: Instructions for building the image if a Dockerfile is available.
- command: The command to run inside the container.
- ports: Port mappings between the host and container.
- volumes: Bind mounts or named volumes for data persistence.
- environment: Environment variables to pass into the container.
- depends_on: Specifies dependencies between services, controlling the start-up order.
Networks
Docker Compose automatically creates a network for your services to communicate. However, you can define custom networks if needed. For example:
yamlnetworks: app-network: driver: bridge
This snippet creates a custom bridge network called app-network
.
Volumes
Volumes are used to persist data between container restarts. You can define named volumes in the volumes
section of the file:
yamlvolumes: db-data:
In this case, db-data
is a named volume that can be mounted to any service.
Running Docker Compose
Once you’ve created your docker-compose.yml
file, running your application is straightforward.
Step 1: Start the Application
Navigate to the directory containing your docker-compose.yml
file and run:
docker-compose up
This command builds, (if necessary), and starts all the services defined in your Docker Compose file. You should see logs from all running containers in your terminal.
Step 2: Run in Detached Mode
If you want to run your containers in the background, use the -d
flag:
docker-compose up -d
This starts your application in detached mode, allowing you to continue using your terminal.
Step 3: View Running Containers
To see a list of running containers, use:
docker-compose ps
This command displays the status, names, and ports of all containers managed by Docker Compose.
Step 4: Stop the Application
To stop and remove the containers, networks, and volumes defined in your Docker Compose file, run:
docker-compose down
This command stops all running services and removes any associated resources, cleaning up your environment.
Advanced Docker Compose Features
Docker Compose also offers advanced features that make it even more powerful.
Scaling Services
You can scale services by specifying the number of container instances to run. For example, to scale the web
service to three instances, use:
docker-compose up --scale web=3
This command creates three instances of the web
service, distributing the load across them.
Override Configuration
Docker Compose allows you to override configurations using additional YAML files. For example, you might have a docker-compose.override.yml
file for development settings. Compose will automatically apply these overrides when you run your application.
Environment Variables
Docker Compose supports environment variable substitution, allowing you to define variables in an .env
file or directly in the shell. For example:
yamlservices: web: image: "nginx:${NGINX_VERSION}"
If NGINX_VERSION
is set to alpine
, Compose will use the nginx:alpine
image.
Running Commands Inside Containers
You can run commands inside running containers using docker-compose exec
. For example, to open a shell inside the web
container, use:
docker-compose exec web sh
This command gives you direct access to the container’s environment, useful for debugging or running administrative commands.
Best Practices for Using Docker Compose
To get the most out of Docker Compose, consider the following best practices:
- Keep It Simple: Start with a minimal configuration and add complexity as needed.
- Use Named Volumes: For persistent data, use named volumes instead of bind mounts.
- Separate Concerns: Use different services for different components of your application (e.g., database, cache, web).
- Version Control Your Compose Files: Store your
docker-compose.yml
and related files in version control for consistency. - Leverage Overrides: Use override files for different environments, such as development, testing, and production.
Conclusion
Docker Compose is an invaluable tool for managing multi-container Docker applications. By simplifying the process of defining, running, and scaling containers, it enables you to focus on developing your application rather than managing infrastructure. Whether you’re working on a small project or a large-scale application, Docker Compose offers the flexibility and power you need.
With this comprehensive guide, you’re now equipped to start using Docker Compose effectively. From basic setup to advanced features, Docker Compose will undoubtedly enhance your containerization workflow, making it more efficient and reliable.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut