Introduction to Python Script: Understanding the Basics
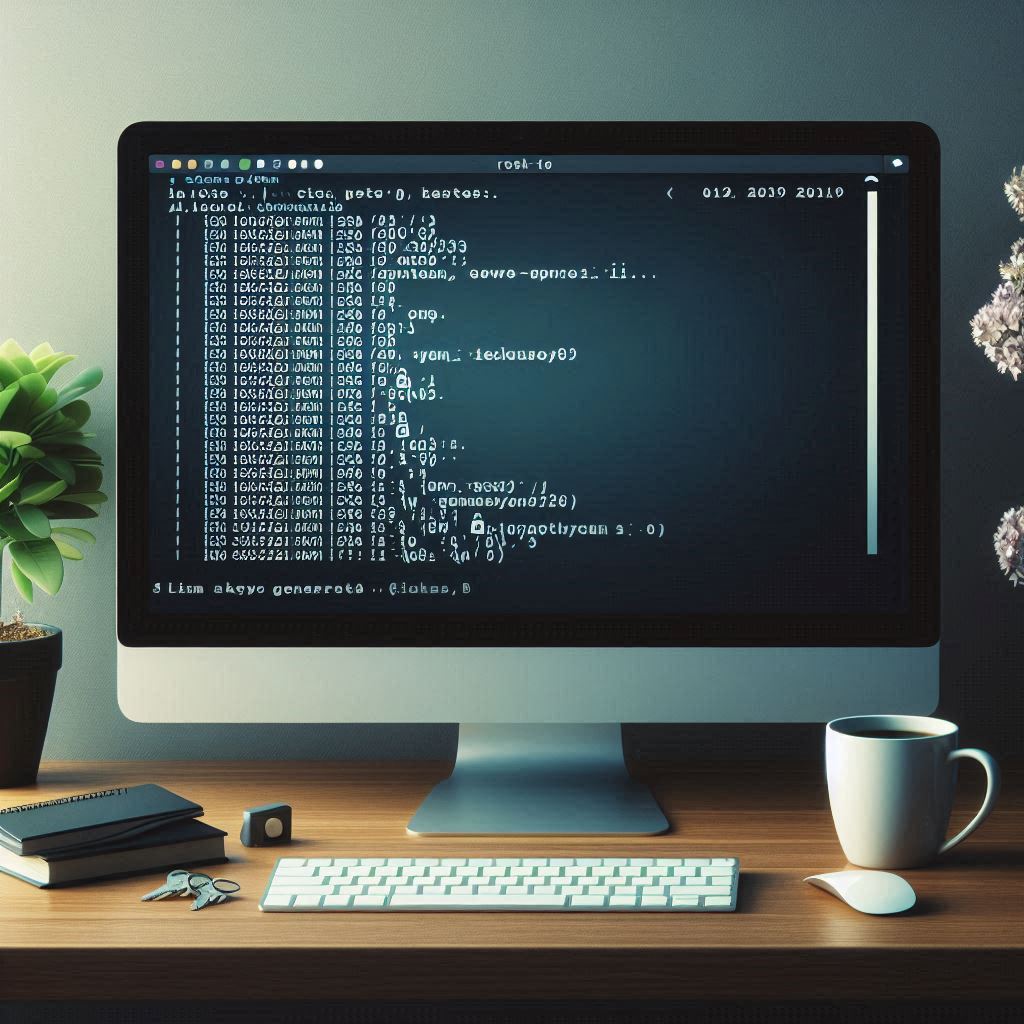
Python is a powerful, high-level programming language that has gained widespread popularity across various fields. It was created by Guido van Rossum and first released in 1991. Python’s design philosophy emphasizes code readability and simplicity, making it an ideal language for beginners and professionals alike. This article will provide a detailed introduction to Python scripting, covering fundamental concepts and essential tools for understanding the basics.
What is Python?
Python is an interpreted language, which means that its code is executed line by line. This feature makes it easy to test and debug. Python is also a high-level language, meaning it abstracts away most of the complex details of the computer’s hardware, allowing developers to focus on programming logic.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This flexibility allows developers to choose the best approach for a given problem. Python is used in various applications, from web development and data analysis to artificial intelligence and automation.
Why Learn Python?
Python’s syntax is designed to be readable and straightforward. This simplicity reduces the learning curve for beginners. Python’s extensive standard library provides modules and functions to handle tasks like file I/O, system calls, and even web browsing. The large community of Python developers has also created numerous libraries and frameworks that simplify complex tasks.
Python is also platform-independent, meaning that Python code can run on any operating system, including Windows, macOS, and Linux. This makes Python a versatile tool for developers in different environments.
Setting Up Python
Before you can start scripting in Python, you need to install the Python interpreter on your computer. The official Python website (python.org) provides installers for various operating systems. After installation, you can check if Python is installed by opening a terminal or command prompt and typing python --version
.
Python comes with an interactive shell, known as IDLE, where you can write and execute Python code. For more complex scripts, you can use a text editor or an Integrated Development Environment (IDE) like PyCharm, VSCode, or Jupyter Notebook.
Writing Your First Python Script
A Python script is simply a file containing Python code. These files typically have a .py
extension. To write your first Python script, open your text editor or IDE and type the following code:
print("Hello, World!")
Save the file as hello.py
and run it from the terminal by typing python hello.py
. This script will output the text “Hello, World!” to the console. This simple program introduces you to Python’s print()
function, which outputs text to the screen.
Understanding Python Syntax
Python’s syntax is designed to be clean and easy to understand. Unlike many other programming languages, Python uses indentation to define code blocks instead of braces {}
or keywords. This feature enforces readable code and reduces errors related to block definitions.
For example, consider the following conditional statement:
if x > 10:
print("x is greater than 10")
else:
print("x is less than or equal to 10")
In this code, the indented lines indicate which statements belong to the if
and else
blocks.
Variables and Data Types
In Python, variables are used to store data that can be used later in the program. You don’t need to declare a variable’s type explicitly; Python infers it from the value you assign.
name = "Alice"
age = 30
height = 5.6
In this example, name
is a string, age
is an integer, and height
is a floating-point number. Python supports several data types, including integers, floats, strings, lists, tuples, sets, and dictionaries.
Lists and Tuples
Lists and tuples are sequences used to store collections of items. A list is mutable, meaning you can change its elements after creation, while a tuple is immutable.
fruits = ["apple", "banana", "cherry"]
coordinates = (10, 20)
You can access elements in a list or tuple using indexing, starting from zero.
print(fruits[0]) # Outputs: apple
print(coordinates[1]) # Outputs: 20
Control Structures
Python provides control structures like conditional statements and loops to control the flow of your program.
Conditional Statements
The if
, elif
, and else
statements allow you to execute code based on conditions.
x = 20
if x > 10:
print("x is greater than 10")
elif x == 10:
print("x is exactly 10")
else:
print("x is less than 10")
Loops
Loops are used to repeat a block of code multiple times. Python provides for
and while
loops.
for i in range(5):
print(i)
This for
loop prints numbers from 0 to 4. The range()
function generates a sequence of numbers.
i = 0
while i < 5:
print(i)
i += 1
his while
loop prints the same sequence of numbers but continues until i
reaches 5.
Functions in Python
Functions are reusable blocks of code that perform a specific task. You define a function using the def
keyword.
def greet(name):
print(f"Hello, {name}!")
You can call this function with a specific argument:
greet("Alice")
This will output: Hello, Alice!
. Functions can also return values using the return
keyword.
def add(a, b):
return a + b
Calling add(5, 3)
will return 8.
Python Modules and Packages
A module is a file containing Python definitions and statements. You can import a module into another script using the import
keyword.
import math
print(math.sqrt(16))
This script imports the math
module and uses its sqrt()
function to calculate the square root of 16.
A package is a collection of modules. Python has a rich ecosystem of packages, and you can install additional ones using a package manager like pip
.
File Handling in Python
Python provides built-in functions for reading from and writing to files. You can open a file using the open()
function.
file = open("example.txt", "w")
file.write("Hello, World!")
file.close()
This code opens a file named example.txt
in write mode, writes a string to it, and then closes the file.
To read from a file, you use a similar approach:
file = open("example.txt", "r")
content = file.read()
print(content)
file.close()
This reads the content of example.txt
and prints it to the console.
Exception Handling
Errors can occur in programs, and Python provides a way to handle these exceptions gracefully. You use try
and except
blocks to catch and handle errors.
try:
x = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
In this example, the ZeroDivisionError
is caught and handled, preventing the program from crashing.
Introduction to Object-Oriented Programming (OOP)
Python supports object-oriented programming, which is a programming paradigm based on the concept of objects. Objects are instances of classes, which can contain both data and functions.
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
print(f"{self.name} says woof!")
my_dog = Dog("Buddy")
my_dog.bark()
In this code, Dog
is a class with a constructor method (__init__
) and a method called bark
. We create an instance of Dog
named Buddy
, and when we call bark()
, it outputs Buddy says woof!
.
Working with Libraries
Python’s power comes from its vast ecosystem of libraries. Libraries like NumPy and pandas are essential for data analysis, while Django and Flask are popular for web development.
To use a library, you need to install it first using pip
, the package installer for Python. For example:
pip install numpy
Once installed, you can import and use the library in your script:
import numpy as np
array = np.array([1, 2, 3])
print(array)
Basic Data Analysis with Python
Python is widely used in data science for analyzing and visualizing data. Libraries like pandas provide powerful data structures for handling datasets, while matplotlib and seaborn allow for creating plots and charts.
Here’s a simple example using pandas:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
This code creates a DataFrame, a table-like data structure, and prints it.
Automation with Python
Python is often used for automating repetitive tasks. For example, you can automate web scraping using libraries like BeautifulSoup or Selenium, or automate file handling and system operations using built-in modules like os
and shutil
.
import os
files = os.listdir("/path/to/directory")
for file in files:
print(file)
This script lists all files in a specified directory.
Getting Help and Learning More
Python has a large and active community, making it easy to find help and resources. The official Python documentation (docs.python.org) is comprehensive and a great starting point for beginners. Additionally, platforms like Stack Overflow, GitHub, and various online tutorials provide valuable learning materials and community support.
Conclusion
Python is a versatile and beginner-friendly programming language that opens up countless possibilities across various fields. By understanding the basics of Python scripting, including variables, data types, control structures, functions, and libraries, you can start building your own programs and exploring more advanced concepts.
The key to mastering Python, like any programming language, is practice. As you write more scripts and solve more problems, you’ll become more comfortable and proficient. Whether you are interested in web development, data analysis, automation, or something else, Python provides the tools you need to achieve your goals.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut