Introduction to Shell Script: Understanding the Basics
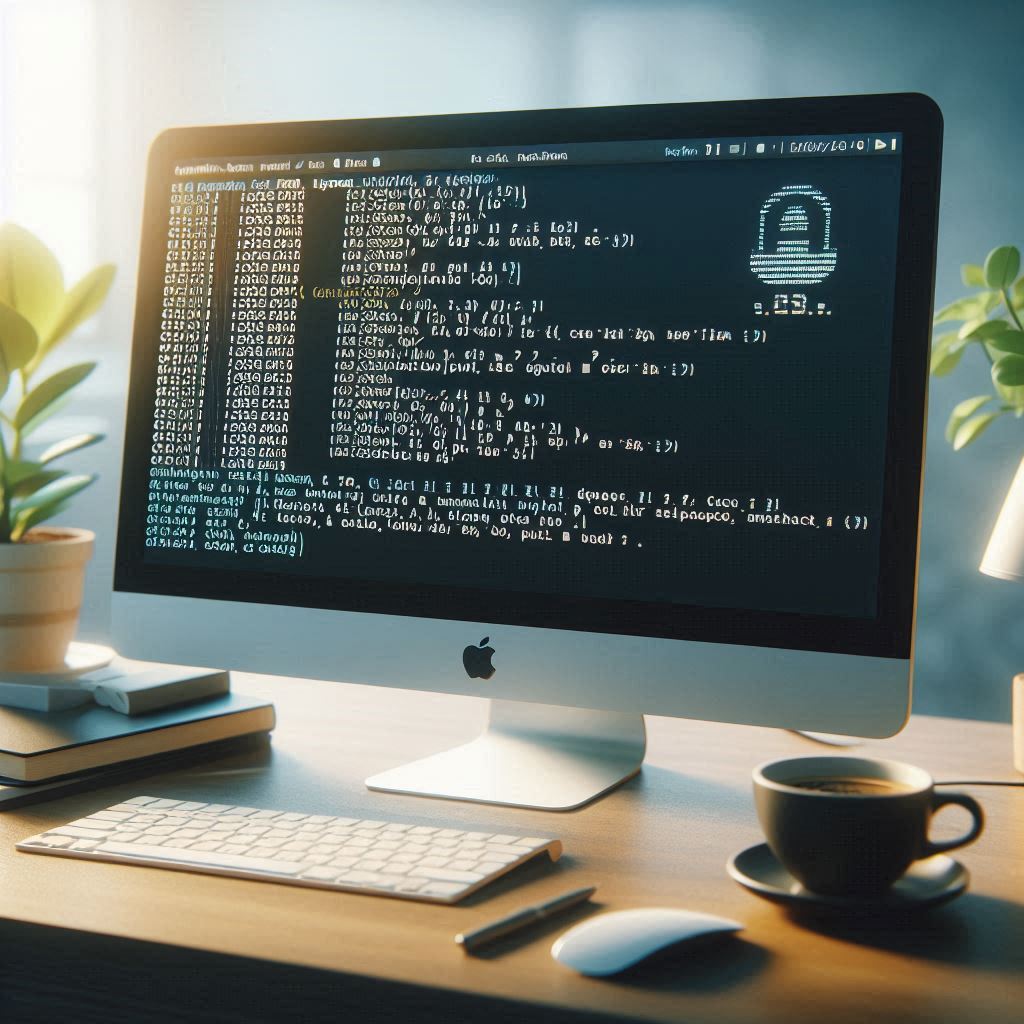
Shell scripting is a powerful tool in the world of computing and software development. It’s a skill that bridges the gap between users and the operating system, allowing for the automation of tasks, the manipulation of files, and the control of system processes. For anyone working in a Linux or UNIX environment, shell scripting is an essential skill. This article will explore what shell scripts are, how they work, and why they are crucial in modern computing.
What is a Shell Script?
A shell script is a program written for the shell, or command line interpreter, of an operating system. Shells are special user interfaces that provide an environment for users to interact with the system. They accept commands from the user, execute them, and return the results. The shell interprets the commands you write, and a shell script is simply a file containing a series of commands.
Types of Shells
There are several types of shells available, each with its unique features and syntax. The most common shells include:
- Bourne Shell (sh): The original UNIX shell, known for its simplicity and efficiency.
- Bourne Again Shell (bash): An enhanced version of the Bourne Shell, widely used due to its versatility and backward compatibility.
- C Shell (csh): This shell offers a syntax similar to the C programming language, which can be advantageous for programmers familiar with C.
- Korn Shell (ksh): Combines features of the Bourne Shell and C Shell, offering robust scripting capabilities.
- Z Shell (zsh): An extended version of bash with additional features, favored by many advanced users.
The Structure of a Shell Script
A shell script typically starts with a “shebang” (#!) followed by the path to the shell interpreter. This line tells the system which shell should be used to execute the script. For example:
bash #!/bin/bash
After the shebang, the script contains a series of commands that the shell interpreter will execute. These commands can include variables, loops, conditionals, and functions.
Basic Components of Shell Scripting
- Variables: Variables store data that can be used and manipulated within the script. In shell scripting, variables are created and used without data types, meaning they can store numbers, strings, or other data types.bashКопиране на код
#!/bin/bash name="John Doe" echo "Hello, $name"
- Loops: Loops allow the repetition of a set of commands. Common loops include
for
,while
, anduntil
.bashКопиране на код#!/bin/bash for i in 1 2 3 4 5 do echo "Number: $i" done
- Conditionals: Conditionals enable decision-making in scripts. The
if-else
structure is commonly used for branching logic.bashКопиране на код#!/bin/bash num=10 if [ $num -gt 5 ] then echo "Number is greater than 5" else echo "Number is less than or equal to 5" fi
- Functions: Functions allow the grouping of commands into reusable blocks. Functions are defined and then called within the script.bashКопиране на код
#!/bin/bash function greet() { echo "Hello, $1" } greet "Alice"
Why Use Shell Scripts?
Shell scripts are used for a variety of reasons, ranging from simple automation tasks to complex system management. Here are some of the key reasons to use shell scripts:
- Automation: Shell scripts can automate repetitive tasks, such as file backups, software installation, and system updates. This saves time and reduces the chance of errors.
- Efficiency: Writing a shell script can be faster and more efficient than performing tasks manually. Scripts can be scheduled to run at specific times, ensuring tasks are completed even when you’re not around.
- Customization: Shell scripts allow users to customize their system environment and workflows to suit specific needs. For instance, you can create custom commands or automate sequences of commands that are often used together.
- System Administration: For system administrators, shell scripts are invaluable. They can manage users, configure network settings, monitor system performance, and perform many other administrative tasks.
- Portability: Shell scripts are text files and can be easily transferred between systems. Most UNIX-based systems come with a shell interpreter, making scripts highly portable across different environments.
Writing Your First Shell Script
Creating a shell script is straightforward. Here’s how you can write and run a simple shell script:
- Open a Text Editor: Use a text editor like
nano
,vi
, or any graphical editor to create a new file. - Write the Script: Start with the shebang, followed by your commands. For example:
#!/bin/bash
echo "This is my first shell script!"
3. Save the Script: Save the file with a .sh
extension, such as my_script.sh
.
4. Make the Script Executable: Before running the script, you need to make it executable. Use the chmod
command:
chmod +x my_script.sh
5. Run the Script: Execute the script by typing ./my_script.sh
in the terminal.
Best Practices in Shell Scripting
- Use Comments: Comments are lines in a script that are not executed. They start with a
#
and are used to explain code. Comments make scripts easier to understand and maintain.
# This script displays a welcome message
echo "Welcome to the shell scripting tutorial!"
2. Error Handling: Include error handling to ensure your script can handle unexpected situations gracefully. Use conditionals to check if commands succeed before proceeding.
if [ $? -eq 0 ]; then
echo "Command succeeded"
else
echo "Command failed"
fi
3. Input Validation: Validate user input to prevent errors and ensure the script behaves as expected. Use read
to capture input and if
statements to validate it.
read -p "Enter your name: " name
if [ -z "$name" ]; then
echo "Name cannot be empty"
exit 1
fi
4. Avoid Hardcoding Values: Instead of hardcoding values, use variables or configuration files. This makes your scripts more flexible and easier to update.
file_path="/path/to/file"
echo "Processing $file_path"
5. Modularize with Functions: Break your script into functions to organize the code better. This makes the script easier to debug and maintain.
function clean_directory() {
rm -rf /path/to/directory/*
echo "Directory cleaned"
}
Common Pitfalls in Shell Scripting
- Misuse of Quotes: Properly use quotes to avoid unexpected behavior. Double quotes allow variable expansion, while single quotes prevent it.
echo "Hello, $name" # Variable expansion
echo 'Hello, $name' # No variable expansion
- Incorrect File Permissions: Always ensure your script has the correct permissions to run. Without executable permission, the script won’t execute.
- Lack of Testing: Test your script in different scenarios to ensure it works as expected. This includes testing with various inputs and conditions.
- Ignoring Return Values: Commands in shell scripts return values that indicate success or failure. Ignoring these can lead to unexpected behavior.
- Overusing Sudo: Avoid using
sudo
inside scripts unless absolutely necessary. Misuse ofsudo
can lead to security risks and unintended system changes.
Advanced Shell Scripting Techniques
- Regular Expressions: Use regular expressions for pattern matching within scripts. This is useful for text processing tasks like searching and replacing.
grep '^a' filename.txt
2. Pipes and Redirection: Use pipes (|
) to send the output of one command as input to another. Redirection (>
, >>
) is used to send output to files.
ls | grep '.txt' > textfiles.txt
3. Process Management: Manage system processes within your scripts using commands like ps
, kill
, and wait
.
process_id=$(pgrep process_name)
kill $process_id
4. Script Arguments: Pass arguments to scripts and use them within the script. This makes your scripts more flexible.
#!/bin/bash
echo "First argument: $1"
echo "Second argument: $2"
5. Interactive Scripts: Make scripts interactive by prompting the user for input and responding to their selections.
echo “Choose an option:”
select option in “Option 1” “Option 2” “Option 3”; do
echo “You chose $option”
break
done
Conclusion
Shell scripting is an invaluable skill for anyone working with UNIX-like operating systems. It empowers users to automate tasks, manage systems, and customize their environment. By understanding the basics, following best practices, and avoiding common pitfalls, you can create efficient and robust shell scripts. Whether you’re a system administrator, developer, or enthusiast, shell scripting opens up a world of possibilities, making your work more efficient and productive. With practice, you’ll be able to harness the full potential of shell scripting, turning complex tasks into simple, repeatable processes.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut