Shells and Shell Scripting
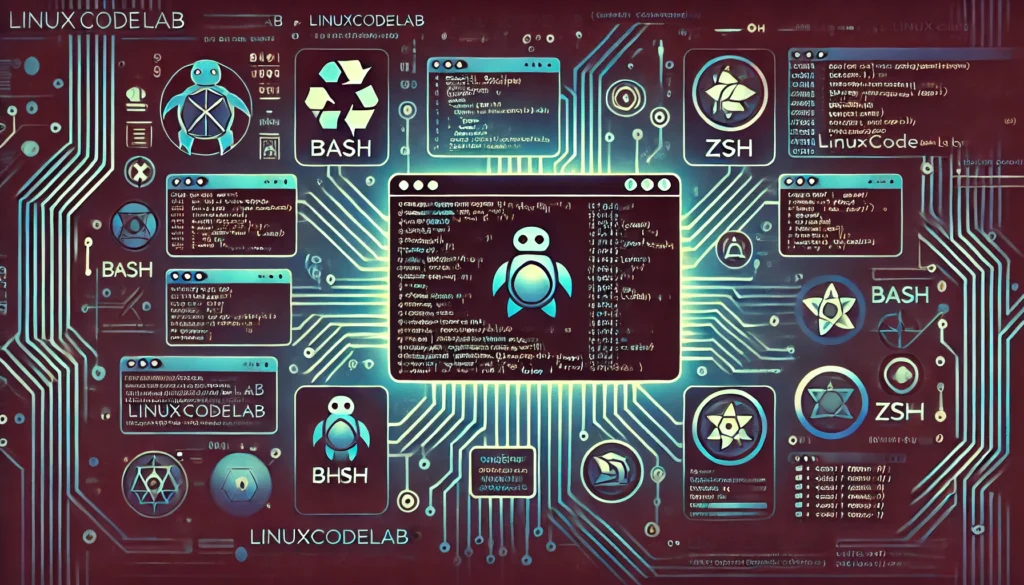
In the world of computing, shells and shell scripting are vital tools that provide users with the ability to interact with operating systems efficiently. Shells serve as an intermediary between the user and the kernel, allowing commands to be executed in a controlled environment. Shell scripting, on the other hand, is a powerful method to automate tasks, streamline workflows, and manipulate data. This article explores the fundamentals of shells, delves into the nuances of shell scripting, and provides practical examples to illustrate key concepts.
Understanding Shells
What is a Shell?
A shell is a command-line interface that allows users to interact with the operating system by typing commands. It acts as a bridge between the user and the system’s core, known as the kernel. When a user inputs a command, the shell interprets it and then communicates with the kernel to execute the command.
Types of Shells
There are several types of shells available, each with its own syntax and features. The most common shells include:
- Bourne Shell (sh): The original Unix shell, known for its simplicity and portability.
- Bourne Again Shell (bash): An enhanced version of the Bourne Shell, widely used in Linux distributions.
- C Shell (csh): Known for its C-like syntax, often used for scripting in older Unix systems.
- Korn Shell (ksh): Combines features of the Bourne Shell and C Shell, offering scripting and interactive use.
- Z Shell (zsh): A highly customizable shell that extends the functionality of the Bourne Again Shell.
Each shell has unique features, but bash is the most commonly used due to its widespread availability and extensive support for scripting.
The Role of the Shell
The primary role of the shell is to interpret user commands and manage the execution of programs. It allows users to navigate the file system, run programs, and manage processes. Additionally, the shell provides features like command history, aliases, and job control, making it a versatile tool for both novice and experienced users.
Shell Scripting
What is Shell Scripting?
Shell scripting is the practice of writing scripts using shell commands to automate tasks. A shell script is a text file containing a series of commands that the shell interprets and executes. These scripts can perform a wide range of functions, from simple file manipulation to complex data processing and system administration tasks.
Why Use Shell Scripting?
Shell scripting offers several advantages:
- Automation: Repetitive tasks can be automated, saving time and reducing errors.
- Efficiency: Scripts can handle complex tasks quickly and efficiently.
- Customization: Users can create tailored solutions for specific problems.
- Portability: Shell scripts can be run on different Unix-like systems with little or no modification.
These benefits make shell scripting an invaluable skill for system administrators, developers, and power users.
Basic Structure of a Shell Script
A basic shell script consists of a series of commands, usually beginning with a shebang (#!
) followed by the path to the shell interpreter. This line indicates which shell should be used to execute the script.
#!/bin/bash # This is a comment echo "Hello, World!"br>
In this example, the script uses bash as the interpreter and outputs the text “Hello, World!” to the terminal. The #
symbol is used for comments, which are ignored by the shell.
Variables in Shell Scripting
Variables in shell scripting are used to store data that can be used and manipulated within the script. Variables do not require declaration and can hold strings, numbers, or even the output of commands.
#!/bin/bash name="Alice" echo "Hello, $name!"br>
Here, the variable name
is assigned the value “Alice”. The script then outputs “Hello, Alice!” by referencing the variable with a $
sign.
Conditional Statements
Conditional statements are used to make decisions within a script. The most common conditional statement is the if
statement, which allows a script to execute commands based on certain conditions.
#!/bin/bash num=10 if [ $num -gt 5 ]; then echo "The number is greater than 5." else echo "The number is 5 or less." fibr>
In this script, the if
statement checks if the variable num
is greater than 5. If the condition is true, it prints a message; otherwise, it prints a different message.
Loops in Shell Scripting
Loops are used to repeat a set of commands multiple times. The two most common types of loops are for
and while
loops.
#!/bin/bash for i in 1 2 3 4 5; do echo "Iteration $i" donebr>
This script uses a for
loop to iterate over a list of numbers and prints a message for each iteration.
Functions in Shell Scripting
Functions allow you to group commands into reusable blocks. Functions can be called multiple times within a script, making code more modular and maintainable.
#!/bin/bash greet() { echo "Hello, $1!" } greet "Bob" greet "Alice"br>
In this example, the greet
function takes an argument and prints a greeting. The function is called twice with different arguments.
Handling Input and Output
Shell scripts often need to handle user input and output. The read
command is used to capture user input, while output can be redirected to files or other commands.
#!/bin/bash echo "Enter your name:" read user_name echo "Hello, $user_name!" > greeting.txtbr>
This script prompts the user for their name and writes a greeting to a file called greeting.txt
.
Error Handling and Debugging
Error handling is crucial in shell scripting to ensure scripts run smoothly. The set -e
command can be used to stop the script if any command returns a non-zero exit status. Additionally, debugging can be done using the -x
option, which prints each command before executing it.
#!/bin/bash set -e cp file.txt /nonexistent_directorybr>
In this script, if the cp
command fails because the directory does not exist, the script will terminate immediately.
Advanced Shell Scripting Techniques
Using Command Substitution
Command substitution allows the output of a command to be used as input for another command or stored in a variable.
#!/bin/bash current_date=$(date) echo "Today's date is $current_date"br>
Here, the date
command’s output is stored in the current_date
variable and then printed.
Processing Text with sed
and awk
sed
and awk
are powerful tools for text processing in shell scripts. sed
is used for stream editing, while awk
is a pattern scanning and processing language.
#!/bin/bash echo "Hello World" | sed 's/World/Shell/'br>
This command uses sed
to replace the word “World” with “Shell” in the output.
Working with Arrays
Shells like bash support arrays, which can store multiple values in a single variable.
#!/bin/bash fruits=("Apple" "Banana" "Cherry") echo "The first fruit is ${fruits[0]}"br>
In this example, an array named fruits
is created, and the first element is accessed and printed.
Conclusion
Shells and shell scripting are essential tools in the arsenal of anyone working with Unix-like operating systems. Shells provide a powerful interface to interact with the system, while shell scripting enables automation and customization of tasks. By mastering the basics, such as variables, loops, and functions, and exploring advanced techniques like command substitution and text processing, users can harness the full potential of shell scripting.
Whether you are a system administrator, developer, or an enthusiast, understanding and utilizing shell scripting can significantly enhance your productivity and efficiency. With practice and experimentation, shell scripting can become an indispensable part of your workflow, enabling you to solve complex problems with elegant and efficient solutions.
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut