Introduction to Python and Programming Basics
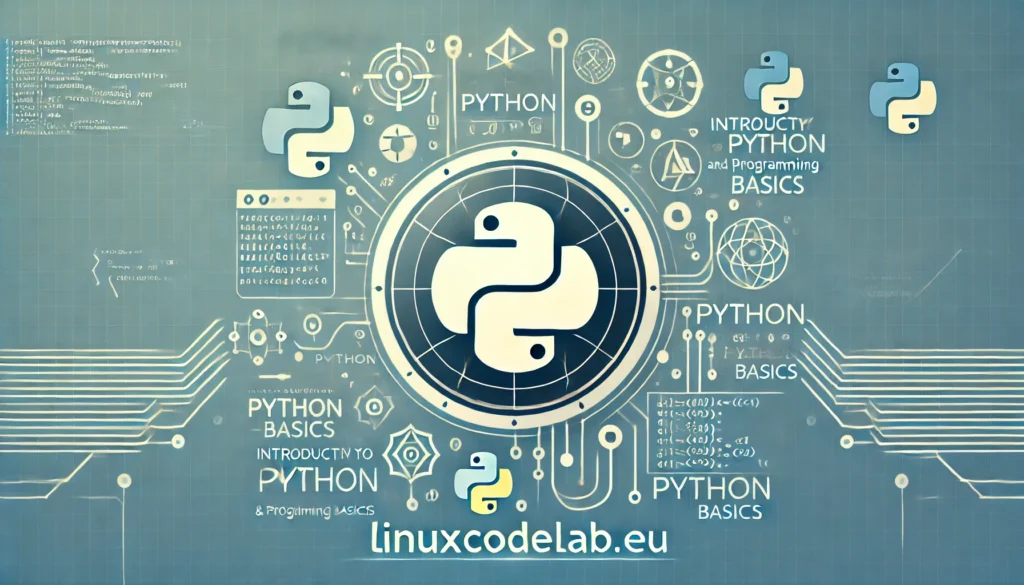
Python has emerged as one of the most popular programming languages today. Known for its simplicity and readability, Python is widely used across various fields, from web development to data analysis. This article will introduce you to Python and cover fundamental programming concepts. Whether you’re a beginner or someone looking to refresh your knowledge, this guide is for you.
What Is Python?
Python is a high-level programming language created by Guido van Rossum in 1991. Unlike low-level languages like C or Assembly, Python abstracts many of the complex details, making it easier for beginners to learn. Python is also an interpreted language, meaning that you can run Python code directly without needing to compile it first.
Python is known for its readable syntax, which closely resembles the English language. This readability makes it easier to understand and write code, especially for beginners. Python’s simplicity does not mean it’s less powerful. It’s widely used in various domains, including web development, data science, machine learning, and automation.
Why Learn Python?
Python’s versatility and ease of use make it an excellent choice for beginners and professionals alike. Here are some reasons to learn Python:
- Ease of Learning: Python’s syntax is straightforward and easy to learn, making it ideal for beginners.
- Versatility: Python can be used for web development, data analysis, automation, and more.
- Community Support: Python has a large and active community, providing a wealth of resources and libraries.
- Career Opportunities: Python is in high demand, and learning it can open doors to various job opportunities.
Installing Python
Before you can start coding in Python, you need to install it on your computer. Here’s how you can do it:
- Download Python: Go to the official Python website (python.org) and download the latest version.
- Install Python: Run the installer and follow the instructions. Make sure to check the option to add Python to your system’s PATH.
- Verify Installation: Open a command prompt or terminal and type
python --version
to verify the installation.
Getting Started with Python Programming
Now that you have Python installed, let’s dive into the basics of programming.
Hello, World!
The “Hello, World!” program is a simple script that outputs “Hello, World!” to the screen. It’s the most basic program you can write in any language.
print("Hello, World!")
When you run this code, Python executes the print()
function and displays “Hello, World!” on the screen. This simple example demonstrates Python’s syntax and how easy it is to start coding.
Variables and Data Types
Variables are used to store data in a program. In Python, you don’t need to declare the type of a variable explicitly; it’s inferred from the value you assign.
name = "Alice" age = 25 height = 5.6
In this example, name
is a string, age
is an integer, and height
is a floating-point number. Python supports several data types, including:
- Integers: Whole numbers (e.g., 1, 42, -7).
- Floats: Decimal numbers (e.g., 3.14, -0.001).
- Strings: Text enclosed in quotes (e.g., “Hello”, ‘Python’).
- Booleans: True or False values (e.g.,
True
,False
).
Basic Operations
Python allows you to perform basic arithmetic operations like addition, subtraction, multiplication, and division.
x = 10 y = 3 # Addition print(x + y) # Outputs: 13 # Subtraction print(x - y) # Outputs: 7 # Multiplication print(x * y) # Outputs: 30 # Division print(x / y) # Outputs: 3.3333...
Python also supports more advanced operations like exponentiation and modulus.
# Exponentiation print(x ** y) # Outputs: 1000 # Modulus print(x % y) # Outputs: 1
Conditional Statements
Conditional statements allow your program to make decisions based on certain conditions. The if
statement is used for this purpose.
age = 18 if age >= 18: print("You are an adult.") else: print("You are a minor.")
In this example, the program checks if age
is greater than or equal to 18. If true, it prints “You are an adult.” Otherwise, it prints “You are a minor.”
Loops
Loops are used to repeat a block of code multiple times. Python supports for
and while
loops.
For Loop:
for i in range(5): print(i)
This loop prints the numbers 0 to 4. The range(5)
function generates a sequence of numbers from 0 to 4.
While Loop:
count = 0 while count < 5: print(count) count += 1
This loop works similarly, but continues until the count
variable reaches 5.
Functions
Functions are reusable blocks of code that perform a specific task. You can define a function using the def
keyword.
def greet(name): print(f"Hello, {name}!") greet("Alice") greet("Bob")
This function, greet
, takes a name
as an argument and prints a greeting. You can call the function with different arguments to reuse the code.
Working with Lists and Dictionaries
Python provides powerful data structures like lists and dictionaries to store collections of data.
Lists
Lists are ordered collections of items, which can be of any data type.
fruits = ["apple", "banana", "cherry"] # Accessing items print(fruits[0]) # Outputs: apple # Modifying items fruits[1] = "blueberry" # Adding items fruits.append("date") # Removing items fruits.remove("cherry")
You can perform various operations on lists, such as accessing, modifying, adding, or removing items.
Dictionaries
Dictionaries store data as key-value pairs. Each key is unique, and it’s used to access the corresponding value.
person = { "name": "Alice", "age": 25, "city": "New York" } # Accessing values print(person["name"]) # Outputs: Alice # Adding a new key-value pair person["email"] = "alice@example.com" # Modifying values person["age"] = 26 # Removing a key-value pair del person["city"]
Dictionaries are useful when you need to associate values with specific keys and retrieve them efficiently.
Introduction to Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of objects. Python supports OOP, allowing you to create classes and objects.
Classes and Objects
A class is a blueprint for creating objects, which are instances of the class. Each object can have attributes (variables) and methods (functions).
class Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): print("Woof!") # Creating an object my_dog = Dog("Buddy", 3) # Accessing attributes and methods print(my_dog.name) # Outputs: Buddy my_dog.bark() # Outputs: Woof!
In this example, Dog
is a class with attributes name
and age
, and a method bark
. The __init__
method initializes the object’s attributes.
Handling Errors
In programming, errors are inevitable. Python provides a way to handle errors gracefully using try-except
blocks.
try: x = 10 / 0 except ZeroDivisionError: print("You cannot divide by zero.")
In this example, the code inside the try
block will raise a ZeroDivisionError
. The except
block catches this error and prints an appropriate message instead of crashing the program.
Introduction to Python Libraries
Python’s strength lies in its extensive collection of libraries. Libraries are collections of pre-written code that you can use to perform common tasks.
Popular Python Libraries
- NumPy: Used for numerical computations.
- Pandas: Ideal for data manipulation and analysis.
- Matplotlib: Great for creating visualizations.
- Requests: Helps in making HTTP requests to interact with web services.
- Django: A powerful framework for web development.
To use a library, you typically need to install it using pip, Python’s package installer, and then import it into your program.
import numpy as np array = np.array([1, 2, 3, 4]) print(array)
Conclusion
Learning Python opens up a world of possibilities in programming. With its simple syntax and powerful capabilities, Python is an excellent choice for beginners and experienced developers alike. This introduction covered the basics, from installing Python to understanding variables, loops, functions, and even object-oriented programming. With practice, you’ll soon be able to write more complex programs and explore Python’s vast libraries.
Keep coding, experimenting, and exploring the endless opportunities that Python offers!
Thank you for reading the article! If you found the information useful, you can donate using the buttons below:
Donate ☕️ with PayPalDonate 💳 with Revolut